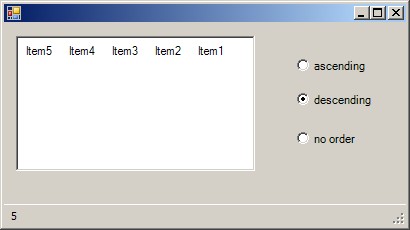
Imports System.Windows.Forms
public class ListViewSorting
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Dim mycount As Integer
mycount = listview1.Items.Count
ToolStripStatusLabel1.Text = Str(mycount)
End Sub
Private Sub ListView1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ListView1.Click
Dim i As Integer
Dim mycount As Integer
mycount = ListView1.Items.Count
For i = 0 To mycount - 1
If ListView1.Items(i).Selected = True Then
ToolStripStatusLabel1.Text = ListView1.Items(i).Text + " selected"
Exit For
End If
Next
End Sub
Private Sub RadioButton1_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButton1.CheckedChanged
ListView1.Sorting = SortOrder.Ascending
End Sub
Private Sub RadioButton2_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButton2.CheckedChanged
ListView1.Sorting = SortOrder.Descending
End Sub
Private Sub RadioButton3_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButton3.CheckedChanged
ListView1.Sorting = SortOrder.None
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.components = New System.ComponentModel.Container
Dim ListViewItem1 As System.Windows.Forms.ListViewItem = New System.Windows.Forms.ListViewItem("Item1", 0)
Dim ListViewItem2 As System.Windows.Forms.ListViewItem = New System.Windows.Forms.ListViewItem("Item2", 1)
Dim ListViewItem3 As System.Windows.Forms.ListViewItem = New System.Windows.Forms.ListViewItem("Item3", 2)
Dim ListViewItem4 As System.Windows.Forms.ListViewItem = New System.Windows.Forms.ListViewItem("Item4", 3)
Dim ListViewItem5 As System.Windows.Forms.ListViewItem = New System.Windows.Forms.ListViewItem("Item5", 4)
Me.ListView1 = New System.Windows.Forms.ListView
Me.RadioButton1 = New System.Windows.Forms.RadioButton
Me.RadioButton2 = New System.Windows.Forms.RadioButton
Me.RadioButton3 = New System.Windows.Forms.RadioButton
Me.StatusStrip1 = New System.Windows.Forms.StatusStrip
Me.ToolStripStatusLabel1 = New System.Windows.Forms.ToolStripStatusLabel
Me.StatusStrip1.SuspendLayout()
Me.SuspendLayout()
'
'ListView1
'
Me.ListView1.Items.AddRange(New System.Windows.Forms.ListViewItem() {ListViewItem1, ListViewItem2, ListViewItem3, ListViewItem4, ListViewItem5})
Me.ListView1.LargeImageList = Me.ImageList1
Me.ListView1.Location = New System.Drawing.Point(16, 15)
Me.ListView1.Margin = New System.Windows.Forms.Padding(4, 4, 4, 4)
Me.ListView1.Name = "ListView1"
Me.ListView1.Size = New System.Drawing.Size(317, 155)
Me.ListView1.TabIndex = 0
Me.ListView1.UseCompatibleStateImageBehavior = False
'
'RadioButton1
'
Me.RadioButton1.AutoSize = True
Me.RadioButton1.Location = New System.Drawing.Point(391, 39)
Me.RadioButton1.Margin = New System.Windows.Forms.Padding(4, 4, 4, 4)
Me.RadioButton1.Name = "RadioButton1"
Me.RadioButton1.Size = New System.Drawing.Size(58, 19)
Me.RadioButton1.TabIndex = 1
Me.RadioButton1.TabStop = True
Me.RadioButton1.Text = "ascending"
Me.RadioButton1.UseVisualStyleBackColor = True
'
'RadioButton2
'
Me.RadioButton2.AutoSize = True
Me.RadioButton2.Location = New System.Drawing.Point(391, 79)
Me.RadioButton2.Margin = New System.Windows.Forms.Padding(4, 4, 4, 4)
Me.RadioButton2.Name = "RadioButton2"
Me.RadioButton2.Size = New System.Drawing.Size(58, 19)
Me.RadioButton2.TabIndex = 2
Me.RadioButton2.TabStop = True
Me.RadioButton2.Text = "descending"
Me.RadioButton2.UseVisualStyleBackColor = True
'
'RadioButton3
'
Me.RadioButton3.AutoSize = True
Me.RadioButton3.Location = New System.Drawing.Point(391, 124)
Me.RadioButton3.Margin = New System.Windows.Forms.Padding(4, 4, 4, 4)
Me.RadioButton3.Name = "RadioButton3"
Me.RadioButton3.Size = New System.Drawing.Size(73, 19)
Me.RadioButton3.TabIndex = 3
Me.RadioButton3.TabStop = True
Me.RadioButton3.Text = "no order"
Me.RadioButton3.UseVisualStyleBackColor = True
'
'StatusStrip1
'
Me.StatusStrip1.Items.AddRange(New System.Windows.Forms.ToolStripItem() {Me.ToolStripStatusLabel1})
Me.StatusStrip1.Location = New System.Drawing.Point(0, 212)
Me.StatusStrip1.Name = "StatusStrip1"
Me.StatusStrip1.Padding = New System.Windows.Forms.Padding(1, 0, 19, 0)
Me.StatusStrip1.Size = New System.Drawing.Size(536, 22)
Me.StatusStrip1.TabIndex = 4
Me.StatusStrip1.Text = "StatusStrip1"
'
'ToolStripStatusLabel1
'
Me.ToolStripStatusLabel1.Name = "ToolStripStatusLabel1"
Me.ToolStripStatusLabel1.Size = New System.Drawing.Size(0, 17)
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(8.0!, 15.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(536, 234)
Me.Controls.Add(Me.StatusStrip1)
Me.Controls.Add(Me.RadioButton3)
Me.Controls.Add(Me.RadioButton2)
Me.Controls.Add(Me.RadioButton1)
Me.Controls.Add(Me.ListView1)
Me.Margin = New System.Windows.Forms.Padding(4, 4, 4, 4)
Me.StatusStrip1.ResumeLayout(False)
Me.StatusStrip1.PerformLayout()
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents ListView1 As System.Windows.Forms.ListView
Friend WithEvents RadioButton1 As System.Windows.Forms.RadioButton
Friend WithEvents RadioButton2 As System.Windows.Forms.RadioButton
Friend WithEvents RadioButton3 As System.Windows.Forms.RadioButton
Friend WithEvents ImageList1 As System.Windows.Forms.ImageList
Friend WithEvents StatusStrip1 As System.Windows.Forms.StatusStrip
Friend WithEvents ToolStripStatusLabel1 As System.Windows.Forms.ToolStripStatusLabel
End Class