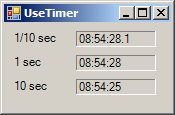
Imports System.Windows.Forms
public class ThreeTimers
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Private Sub tmrTenthSecond_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles tmrTenthSecond.Tick
lblTenthSecond.Text = Now.ToString("hh:mm:ss.f")
End Sub
Private Sub tmrSecond_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles tmrSecond.Tick
lblSeconds.Text = Now.ToString("hh:mm:ss")
End Sub
Private Sub tmrTenSeconds_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles tmrTenSeconds.Tick
lblTenSeconds.Text = Now.ToString("hh:mm:ss")
End Sub
' Display initial times.
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
lblTenthSecond.Text = Now.ToString("hh:mm:ss.f")
lblSeconds.Text = Now.ToString("hh:mm:ss")
lblTenSeconds.Text = Now.ToString("hh:mm:ss")
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.components = New System.ComponentModel.Container
Me.tmrTenthSecond = New System.Windows.Forms.Timer(Me.components)
Me.tmrSecond = New System.Windows.Forms.Timer(Me.components)
Me.tmrTenSeconds = New System.Windows.Forms.Timer(Me.components)
Me.Label1 = New System.Windows.Forms.Label
Me.Label2 = New System.Windows.Forms.Label
Me.Label3 = New System.Windows.Forms.Label
Me.lblTenthSecond = New System.Windows.Forms.Label
Me.lblSeconds = New System.Windows.Forms.Label
Me.lblTenSeconds = New System.Windows.Forms.Label
Me.SuspendLayout()
'
'tmrTenthSecond
'
Me.tmrTenthSecond.Enabled = True
'
'tmrSecond
'
Me.tmrSecond.Enabled = True
Me.tmrSecond.Interval = 1000
'
'tmrTenSeconds
'
Me.tmrTenSeconds.Enabled = True
Me.tmrTenSeconds.Interval = 10000
'
'Label1
'
Me.Label1.AutoSize = True
Me.Label1.Location = New System.Drawing.Point(8, 8)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(46, 13)
Me.Label1.TabIndex = 0
Me.Label1.Text = "1/10 sec"
'
'Label2
'
Me.Label2.AutoSize = True
Me.Label2.Location = New System.Drawing.Point(8, 32)
Me.Label2.Name = "Label2"
Me.Label2.Size = New System.Drawing.Size(29, 13)
Me.Label2.TabIndex = 1
Me.Label2.Text = "1 sec"
'
'Label3
'
Me.Label3.AutoSize = True
Me.Label3.Location = New System.Drawing.Point(8, 56)
Me.Label3.Name = "Label3"
Me.Label3.Size = New System.Drawing.Size(35, 13)
Me.Label3.TabIndex = 2
Me.Label3.Text = "10 sec"
'
'lblTenthSecond
'
Me.lblTenthSecond.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.lblTenthSecond.Location = New System.Drawing.Point(72, 8)
Me.lblTenthSecond.Name = "lblTenthSecond"
Me.lblTenthSecond.Size = New System.Drawing.Size(80, 16)
Me.lblTenthSecond.TabIndex = 3
'
'lblSeconds
'
Me.lblSeconds.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.lblSeconds.Location = New System.Drawing.Point(72, 32)
Me.lblSeconds.Name = "lblSeconds"
Me.lblSeconds.Size = New System.Drawing.Size(80, 16)
Me.lblSeconds.TabIndex = 4
'
'lblTenSeconds
'
Me.lblTenSeconds.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D
Me.lblTenSeconds.Location = New System.Drawing.Point(72, 56)
Me.lblTenSeconds.Name = "lblTenSeconds"
Me.lblTenSeconds.Size = New System.Drawing.Size(80, 16)
Me.lblTenSeconds.TabIndex = 5
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(167, 88)
Me.Controls.Add(Me.lblTenSeconds)
Me.Controls.Add(Me.lblSeconds)
Me.Controls.Add(Me.lblTenthSecond)
Me.Controls.Add(Me.Label3)
Me.Controls.Add(Me.Label2)
Me.Controls.Add(Me.Label1)
Me.Name = "Form1"
Me.Text = "UseTimer"
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents tmrTenthSecond As System.Windows.Forms.Timer
Friend WithEvents tmrSecond As System.Windows.Forms.Timer
Friend WithEvents tmrTenSeconds As System.Windows.Forms.Timer
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents Label2 As System.Windows.Forms.Label
Friend WithEvents Label3 As System.Windows.Forms.Label
Friend WithEvents lblTenthSecond As System.Windows.Forms.Label
Friend WithEvents lblSeconds As System.Windows.Forms.Label
Friend WithEvents lblTenSeconds As System.Windows.Forms.Label
End Class