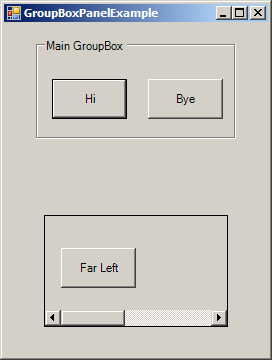
Imports System.Windows.Forms
public class GroupBoxPanel
public Shared Sub Main
Application.Run(New FrmGroupBox)
End Sub
End class
Public Class FrmGroupBox
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Friend WithEvents mainGroupBox As System.Windows.Forms.GroupBox
Private WithEvents mainPanel As System.Windows.Forms.Panel
Friend WithEvents cmdRight As System.Windows.Forms.Button
Friend WithEvents cmdHi As System.Windows.Forms.Button
Friend WithEvents cmdLeft As System.Windows.Forms.Button
Friend WithEvents cmdBye As System.Windows.Forms.Button
Friend WithEvents lblMessage As System.Windows.Forms.Label
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.cmdRight = New System.Windows.Forms.Button()
Me.cmdBye = New System.Windows.Forms.Button()
Me.mainPanel = New System.Windows.Forms.Panel()
Me.cmdLeft = New System.Windows.Forms.Button()
Me.cmdHi = New System.Windows.Forms.Button()
Me.lblMessage = New System.Windows.Forms.Label()
Me.mainGroupBox = New System.Windows.Forms.GroupBox()
Me.mainPanel.SuspendLayout()
Me.mainGroupBox.SuspendLayout()
Me.SuspendLayout()
'
'cmdRight
'
Me.cmdRight.Location = New System.Drawing.Point(352, 32)
Me.cmdRight.Name = "cmdRight"
Me.cmdRight.Size = New System.Drawing.Size(75, 40)
Me.cmdRight.TabIndex = 0
Me.cmdRight.Text = "Far Right"
'
'cmdBye
'
Me.cmdBye.Location = New System.Drawing.Point(112, 40)
Me.cmdBye.Name = "cmdBye"
Me.cmdBye.Size = New System.Drawing.Size(75, 40)
Me.cmdBye.TabIndex = 1
Me.cmdBye.Text = "Bye"
'
'mainPanel
'
Me.mainPanel.AutoScroll = True
Me.mainPanel.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle
Me.mainPanel.Controls.AddRange(New System.Windows.Forms.Control() {Me.cmdLeft, Me.cmdRight})
Me.mainPanel.Location = New System.Drawing.Point(40, 192)
Me.mainPanel.Name = "mainPanel"
Me.mainPanel.Size = New System.Drawing.Size(184, 112)
Me.mainPanel.TabIndex = 1
'
'cmdLeft
'
Me.cmdLeft.Location = New System.Drawing.Point(16, 32)
Me.cmdLeft.Name = "cmdLeft"
Me.cmdLeft.Size = New System.Drawing.Size(75, 40)
Me.cmdLeft.TabIndex = 1
Me.cmdLeft.Text = "Far Left"
'
'cmdHi
'
Me.cmdHi.Location = New System.Drawing.Point(16, 40)
Me.cmdHi.Name = "cmdHi"
Me.cmdHi.Size = New System.Drawing.Size(75, 40)
Me.cmdHi.TabIndex = 0
Me.cmdHi.Text = "Hi"
'
'lblMessage
'
Me.lblMessage.Font = New System.Drawing.Font("Microsoft Sans Serif", 11.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.lblMessage.Location = New System.Drawing.Point(32, 136)
Me.lblMessage.Name = "lblMessage"
Me.lblMessage.Size = New System.Drawing.Size(200, 40)
Me.lblMessage.TabIndex = 2
Me.lblMessage.TextAlign = System.Drawing.ContentAlignment.MiddleCenter
'
'mainGroupBox
'
Me.mainGroupBox.Controls.AddRange(New System.Windows.Forms.Control() {Me.cmdBye, Me.cmdHi})
Me.mainGroupBox.Location = New System.Drawing.Point(32, 16)
Me.mainGroupBox.Name = "mainGroupBox"
Me.mainGroupBox.TabIndex = 0
Me.mainGroupBox.TabStop = False
Me.mainGroupBox.Text = "Main GroupBox"
'
'FrmGroupBox
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(264, 333)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.lblMessage, Me.mainPanel, Me.mainGroupBox})
Me.Name = "FrmGroupBox"
Me.Text = "GroupBoxPanelExample"
Me.mainPanel.ResumeLayout(False)
Me.mainGroupBox.ResumeLayout(False)
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub cmdHi_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles cmdHi.Click
lblMessage.Text = "Hi pressed"
End Sub
Private Sub cmdBye_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles cmdBye.Click
lblMessage.Text = "Bye pressed"
End Sub
Private Sub cmdLeft_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles cmdLeft.Click
lblMessage.Text = "Far left pressed"
End Sub
Private Sub cmdRight_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles cmdRight.Click
lblMessage.Text = "Far right pressed"
End Sub
End Class