Add and remove menu item for File Menu (Recent file list menuitem) : Dynamic Menu « GUI Applications « VB.Net Tutorial
- VB.Net Tutorial
- GUI Applications
- Dynamic Menu
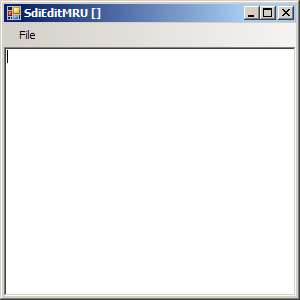
'Visual Basic 2005 Programmer's Reference
'by Rod Stephens (Author)
'# Publisher: Wrox (October 21, 2005)
'# Language: English
'# ISBN-10: 0764571982
'# ISBN-13: 978-0764571985
Imports System.Windows.Forms
Imports System.IO
public class DynamicMenuItemForFileMenu
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class MruList
Private m_ApplicationName As String
Private m_FileMenu As ToolStripMenuItem
Private m_NumEntries As Integer
Private m_FileNames As Collection
Private m_MenuItems As Collection
Public Event OpenFile(ByVal file_name As String)
Public Sub New(ByVal application_name As String, ByVal file_menu As ToolStripMenuItem, ByVal num_entries As Integer)
m_ApplicationName = application_name
m_FileMenu = file_menu
m_NumEntries = num_entries
m_FileNames = New Collection
m_MenuItems = New Collection
' Load saved file names from the Registry.
LoadMruList()
' Display the MRU list.
DisplayMruList()
End Sub
' Load previously saved file names from the Registry.
Private Sub LoadMruList()
Dim file_name As String
For i As Integer = 1 To m_NumEntries
' Get the next file name and title.
file_name = GetSetting(m_ApplicationName, _
"MruList", "FileName" & i, "")
' See if we got anything.
If file_name.Length > 0 Then
' Save this file name.
m_FileNames.Add(file_name, file_name)
End If
Next i
End Sub
' Save the MRU list into the Registry.
Private Sub SaveMruList()
' Remove previous entries.
If GetSetting(m_ApplicationName, "MruList", _
"FileName1", "").Length > 0 Then
DeleteSetting(m_ApplicationName, "MruList")
End If
' Make the new entries.
For i As Integer = 1 To m_FileNames.Count
SaveSetting(m_ApplicationName, _
"MruList", "FileName" & i, _
m_FileNames(i).ToString)
Next i
End Sub
' Display the MRU list.
Private Sub DisplayMruList()
' Remove old menu items from the File menu.
For Each mnu As ToolStripItem In m_MenuItems
m_FileMenu.DropDownItems.Remove(mnu)
Next mnu
m_MenuItems = New Collection
' See if we have any file names.
If m_FileNames.Count > 0 Then
' Make the separator.
Dim sep As New ToolStripSeparator()
m_MenuItems.Add(sep)
m_FileMenu.DropDownItems.Add(sep)
' Make the other menu items.
Dim mnu As ToolStripMenuItem
For i As Integer = 1 To m_FileNames.Count
mnu = New ToolStripMenuItem()
mnu.Text = "&" & i & " " & _
New FileInfo(m_FileNames(i).ToString).Name
AddHandler mnu.Click, AddressOf MruItem_Click
m_MenuItems.Add(mnu)
m_FileMenu.DropDownItems.Add(mnu)
Next i
End If
End Sub
' MRU menu item event handler.
Private Sub MruItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs)
Dim mnu As ToolStripMenuItem
mnu = DirectCast(sender, ToolStripMenuItem)
' Find the menu item that raised this event.
For i As Integer = 1 To m_FileNames.Count
' See if this is the item. (Add 1 for the separator.)
If m_MenuItems(i + 1) Is mnu Then
' This is the item. Raise the OpenFile
' event for its file name.
RaiseEvent OpenFile(m_FileNames(i).ToString)
Exit For
End If
Next i
End Sub
' Add a file to the MRU list.
Public Sub Add(ByVal file_name As String)
' Remove this file from the MRU list
' if it is present.
Dim i As Integer = FileNameIndex(file_name)
If i > 0 Then m_FileNames.Remove(i)
' Add the item to the begining of the list.
If m_FileNames.Count > 0 Then
m_FileNames.Add(file_name, file_name, m_FileNames.Item(1))
Else
m_FileNames.Add(file_name, file_name)
End If
' If the list is too long, remove the last item.
If m_FileNames.Count > m_NumEntries Then
m_FileNames.Remove(m_NumEntries + 1)
End If
' Display the list.
DisplayMruList()
' Save the updated list.
SaveMruList()
End Sub
' Return the index of this file in the list.
Private Function FileNameIndex(ByVal file_name As String) As Integer
For i As Integer = 1 To m_FileNames.Count
If m_FileNames(i).ToString = file_name Then Return i
Next i
Return 0
End Function
' Remove a file from the MRU list.
Public Sub Remove(ByVal file_name As String)
' See if the file is present.
Dim i As Integer = FileNameIndex(file_name)
If i > 0 Then
' Remove the File.
m_FileNames.Remove(i)
' Display the list.
DisplayMruList()
' Save the updated list.
SaveMruList()
End If
End Sub
End Class
Public Class Form1
Private WithEvents m_MruList As MruList
' Initialize the MRU list.
Private Sub Form1_Load(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles Me.Load
m_MruList = New MruList("SdiMruList", mnuFile, 4)
End Sub
' Let the user open a file.
Private Sub mnuFileOpen_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuFileOpen.Click
If dlgOpen.ShowDialog() = Windows.Forms.DialogResult.OK Then
OpenFile(dlgOpen.FileName)
End If
End Sub
' Open a file selected from the MRU list.
Private Sub m_MruList_OpenFile(ByVal file_name As String) _
Handles m_MruList.OpenFile
OpenFile(file_name)
End Sub
' Open a file and add it to the MRU list.
Private Sub OpenFile(ByVal file_name As String)
txtContents.Text = File.ReadAllText(file_name)
txtContents.Select(0, 0)
m_MruList.Add(file_name)
Me.Text = "SdiEditMRU [" & New FileInfo(file_name).Name & "]"
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.MenuStrip1 = New System.Windows.Forms.MenuStrip
Me.mnuFile = New System.Windows.Forms.ToolStripMenuItem
Me.mnuFileOpen = New System.Windows.Forms.ToolStripMenuItem
Me.txtContents = New System.Windows.Forms.TextBox
Me.dlgOpen = New System.Windows.Forms.OpenFileDialog
Me.MenuStrip1.SuspendLayout()
Me.SuspendLayout()
'
'MenuStrip1
'
Me.MenuStrip1.Items.AddRange(New System.Windows.Forms.ToolStripItem() {Me.mnuFile})
Me.MenuStrip1.Location = New System.Drawing.Point(0, 0)
Me.MenuStrip1.Name = "MenuStrip1"
Me.MenuStrip1.Size = New System.Drawing.Size(292, 24)
Me.MenuStrip1.TabIndex = 9
Me.MenuStrip1.Text = "MenuStrip1"
'
'mnuFile
'
Me.mnuFile.DropDownItems.AddRange(New System.Windows.Forms.ToolStripItem() {Me.mnuFileOpen})
Me.mnuFile.Name = "mnuFile"
Me.mnuFile.Size = New System.Drawing.Size(44, 20)
Me.mnuFile.Text = "&File"
'
'mnuFileOpen
'
Me.mnuFileOpen.Name = "mnuFileOpen"
Me.mnuFileOpen.ShortcutKeys = CType((System.Windows.Forms.Keys.Control Or System.Windows.Forms.Keys.O), System.Windows.Forms.Keys)
Me.mnuFileOpen.Size = New System.Drawing.Size(168, 22)
Me.mnuFileOpen.Text = "&Open"
'
'txtContents
'
Me.txtContents.Anchor = CType((((System.Windows.Forms.AnchorStyles.Top Or System.Windows.Forms.AnchorStyles.Bottom) _
Or System.Windows.Forms.AnchorStyles.Left) _
Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.txtContents.Location = New System.Drawing.Point(0, 24)
Me.txtContents.Multiline = True
Me.txtContents.Name = "txtContents"
Me.txtContents.Size = New System.Drawing.Size(292, 249)
Me.txtContents.TabIndex = 8
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.Add(Me.txtContents)
Me.Controls.Add(Me.MenuStrip1)
Me.Name = "Form1"
Me.Text = "SdiEditMRU []"
Me.MenuStrip1.ResumeLayout(False)
Me.MenuStrip1.PerformLayout()
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents MenuStrip1 As System.Windows.Forms.MenuStrip
Friend WithEvents mnuFile As System.Windows.Forms.ToolStripMenuItem
Friend WithEvents mnuFileOpen As System.Windows.Forms.ToolStripMenuItem
Friend WithEvents txtContents As System.Windows.Forms.TextBox
Friend WithEvents dlgOpen As System.Windows.Forms.OpenFileDialog
End Class
15.6.Dynamic Menu |
| 15.6.1. | Add and remove menu item for File Menu (Recent file list menuitem) | 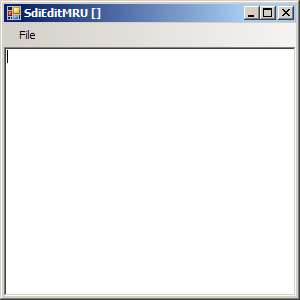 |