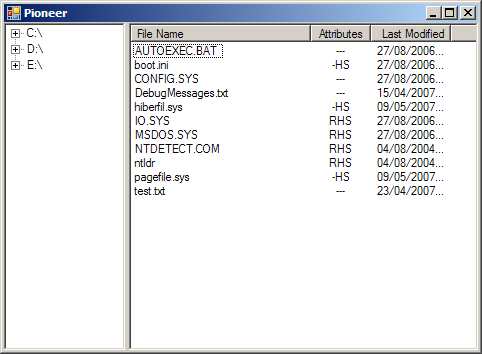
'Sams Teach Yourself Visual Basic .NET in 21 Days
'By Lowell Mauer
'Published 2001
'Sams Publishing
'ISBN 0672322714
Imports System.IO
Imports System.Windows.Forms
public class TreeViewFileFolderFileAttribute
public Shared Sub Main
Application.Run(New PioneerForm)
End Sub
End class
Public Class PioneerForm
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Friend WithEvents tvwFolders As System.Windows.Forms.TreeView
Friend WithEvents splView As System.Windows.Forms.Splitter
Friend WithEvents lvwFiles As System.Windows.Forms.ListView
Friend WithEvents hdrName As System.Windows.Forms.ColumnHeader
Friend WithEvents hdrModified As System.Windows.Forms.ColumnHeader
Friend WithEvents hdrAttributes As System.Windows.Forms.ColumnHeader
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.splView = New System.Windows.Forms.Splitter()
Me.lvwFiles = New System.Windows.Forms.ListView()
Me.tvwFolders = New System.Windows.Forms.TreeView()
Me.hdrName = New System.Windows.Forms.ColumnHeader()
Me.hdrAttributes = New System.Windows.Forms.ColumnHeader()
Me.hdrModified = New System.Windows.Forms.ColumnHeader()
Me.SuspendLayout()
'
'splView
'
Me.splView.Location = New System.Drawing.Point(121, 0)
Me.splView.Name = "splView"
Me.splView.Size = New System.Drawing.Size(4, 327)
Me.splView.TabIndex = 1
Me.splView.TabStop = False
'
'lvwFiles
'
Me.lvwFiles.Columns.AddRange(New System.Windows.Forms.ColumnHeader() {Me.hdrName, Me.hdrAttributes, Me.hdrModified})
Me.lvwFiles.Dock = System.Windows.Forms.DockStyle.Fill
Me.lvwFiles.Location = New System.Drawing.Point(125, 0)
Me.lvwFiles.Name = "lvwFiles"
Me.lvwFiles.Size = New System.Drawing.Size(349, 327)
Me.lvwFiles.TabIndex = 2
Me.lvwFiles.View = System.Windows.Forms.View.Details
'
'tvwFolders
'
Me.tvwFolders.Dock = System.Windows.Forms.DockStyle.Left
Me.tvwFolders.ImageIndex = -1
Me.tvwFolders.Name = "tvwFolders"
Me.tvwFolders.SelectedImageIndex = -1
Me.tvwFolders.Size = New System.Drawing.Size(121, 327)
Me.tvwFolders.TabIndex = 0
'
'hdrName
'
Me.hdrName.Text = "File Name"
Me.hdrName.Width = 180
'
'hdrAttributes
'
Me.hdrAttributes.Text = "Attributes"
Me.hdrAttributes.TextAlign = System.Windows.Forms.HorizontalAlignment.Center
'
'hdrModified
'
Me.hdrModified.Text = "Last Modified"
Me.hdrModified.TextAlign = System.Windows.Forms.HorizontalAlignment.Right
Me.hdrModified.Width = 80
'
'PioneerForm
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(474, 327)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.lvwFiles, Me.splView, Me.tvwFolders})
Me.Name = "PioneerForm"
Me.Text = "Pioneer"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub PioneerForm_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) _
Handles MyBase.Load
'add drives to the treeview
Dim sDrives() As String = Directory.GetLogicalDrives()
Dim sDrive As String
Dim tvwNode As TreeNode
For Each sDrive In sDrives
tvwNode = tvwFolders.Nodes.Add(sDrive)
'add a dummy node
tvwNode.Nodes.Add("dummy")
Next
End Sub
Private Sub tvwFolders_BeforeExpand(ByVal sender As Object, _
ByVal e As System.Windows.Forms.TreeViewCancelEventArgs) _
Handles tvwFolders.BeforeExpand
'see if we already know what the children are
' (we don't if there is still a dummy there)
Dim oNode As TreeNode = CType(e.Node, TreeNode)
If oNode.Nodes(0).Text = "dummy" Then
'remove the dummy
oNode.Nodes(0).Remove()
'add the real children
GetChildren(oNode)
End If
End Sub
Private Sub tvwFolders_AfterSelect(ByVal sender As Object, _
ByVal e As System.Windows.Forms.TreeViewEventArgs) _
Handles tvwFolders.AfterSelect
Dim sFiles() As String = Directory.GetFiles(tvwFolders.SelectedNode.FullPath)
Dim sFile As String
Dim oItem As ListViewItem
lvwFiles.Items.Clear()
For Each sFile In sFiles
oItem = lvwFiles.Items.Add(StripPath(sFile))
If lvwFiles.Items.Count > 0 Then
oItem.SubItems.Add(GetAttributeString(sFile))
oItem.SubItems.Add(File.GetLastWriteTime(sFile))
End If
Next
End Sub
Private Sub GetChildren(ByVal node As TreeNode)
Dim sDirs() As String = Directory.GetDirectories(node.FullPath)
Dim sDir As String
Dim oNode As TreeNode
For Each sDir In sDirs
oNode = node.Nodes.Add(StripPath(sDir))
'add a dummy node as a child
oNode.Nodes.Add("dummy")
Next
End Sub
Private Function GetAttributeString(ByVal fileName As String) As String
Dim sReturn As String
Dim oAttr As FileAttributes = File.GetAttributes(fileName)
If (oAttr And FileAttributes.ReadOnly) = FileAttributes.ReadOnly Then
sReturn = "R"
Else
sReturn = "-"
End If
If (oAttr And FileAttributes.Hidden) = FileAttributes.Hidden Then
sReturn += "H"
Else
sReturn += "-"
End If
If (oAttr And FileAttributes.System) = FileAttributes.System Then
sReturn += "S"
Else
sReturn += "-"
End If
Return sReturn
End Function
Private Function StripPath(ByVal path As String) As String
'removes the leading path from the filename
Dim iPos As Integer
'find the last \ character
iPos = path.LastIndexOf("\")
'everything after it is the actual filename
Return path.Substring(iPos + 1)
End Function
End Class