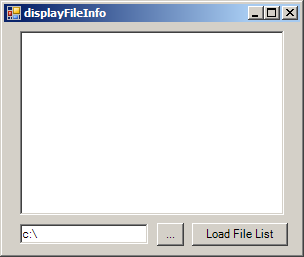
Imports System
Imports System.IO
Imports System.Windows.Forms
public class DisplayFileInfoExplore
public Shared Sub Main
Application.Run(New displayFileInfo)
End Sub
End class
Public Class displayFileInfo
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents pathToLoadFrom As System.Windows.Forms.TextBox
Friend WithEvents loadFileList As System.Windows.Forms.Button
Friend WithEvents browseForFolder As System.Windows.Forms.Button
Friend WithEvents browseDialog As System.Windows.Forms.FolderBrowserDialog
Friend WithEvents fileList As System.Windows.Forms.TreeView
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.pathToLoadFrom = New System.Windows.Forms.TextBox
Me.loadFileList = New System.Windows.Forms.Button
Me.browseForFolder = New System.Windows.Forms.Button
Me.browseDialog = New System.Windows.Forms.FolderBrowserDialog
Me.fileList = New System.Windows.Forms.TreeView
Me.SuspendLayout()
'
'pathToLoadFrom
'
Me.pathToLoadFrom.Anchor = CType(((System.Windows.Forms.AnchorStyles.Bottom Or System.Windows.Forms.AnchorStyles.Left) _
Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.pathToLoadFrom.Location = New System.Drawing.Point(16, 201)
Me.pathToLoadFrom.Name = "pathToLoadFrom"
Me.pathToLoadFrom.Size = New System.Drawing.Size(128, 20)
Me.pathToLoadFrom.TabIndex = 1
Me.pathToLoadFrom.Text = "c:\"
'
'loadFileList
'
Me.loadFileList.Anchor = CType((System.Windows.Forms.AnchorStyles.Bottom Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.loadFileList.Location = New System.Drawing.Point(188, 200)
Me.loadFileList.Name = "loadFileList"
Me.loadFileList.Size = New System.Drawing.Size(96, 23)
Me.loadFileList.TabIndex = 2
Me.loadFileList.Text = "Load File List"
'
'browseForFolder
'
Me.browseForFolder.Anchor = CType((System.Windows.Forms.AnchorStyles.Bottom Or System.Windows.Forms.AnchorStyles.Right), System.Windows.Forms.AnchorStyles)
Me.browseForFolder.Location = New System.Drawing.Point(153, 200)
Me.browseForFolder.Name = "browseForFolder"
Me.browseForFolder.Size = New System.Drawing.Size(27, 23)
Me.browseForFolder.TabIndex = 3
Me.browseForFolder.Text = "..."
'
'fileList
'
Me.fileList.ImageIndex = -1
Me.fileList.Location = New System.Drawing.Point(16, 8)
Me.fileList.Name = "fileList"
Me.fileList.SelectedImageIndex = -1
Me.fileList.Size = New System.Drawing.Size(264, 184)
Me.fileList.TabIndex = 4
'
'FileInfo
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(296, 230)
Me.Controls.Add(Me.fileList)
Me.Controls.Add(Me.browseForFolder)
Me.Controls.Add(Me.loadFileList)
Me.Controls.Add(Me.pathToLoadFrom)
Me.Name = "FileInfo"
Me.Text = "displayFileInfo"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub browseForFolder_Click( _
ByVal sender As System.Object, _
ByVal e As System.EventArgs) _
Handles browseForFolder.Click
If IO.Directory.Exists(pathToLoadFrom.Text) Then
Me.browseDialog.SelectedPath = Me.pathToLoadFrom.Text
End If
If Me.browseDialog.ShowDialog = DialogResult.OK Then
Me.pathToLoadFrom.Text = Me.browseDialog.SelectedPath
End If
End Sub
Private Sub loadFileList_Click( _
ByVal sender As System.Object, _
ByVal e As System.EventArgs) _
Handles loadFileList.Click
GetFileList(Me.pathToLoadFrom.Text)
End Sub
Public Overloads Sub GetFileList(ByVal sourcePath As String)
Dim nodes As TreeNodeCollection = Me.fileList.Nodes
GetFileList(sourcePath, nodes)
End Sub
Public Overloads Sub GetFileList( _
ByVal sourcePath As String, _
ByVal nodes As TreeNodeCollection)
nodes.Clear()
Try
For Each d As String In _
Directory.GetDirectories(sourcePath)
Dim dInfo As New DirectoryInfo(d)
Dim dirNode As New DirectoryTreeNode(dInfo)
nodes.Add(dirNode)
GetFileList(Path.Combine(sourcePath, d), _
dirNode.Nodes)
Next
For Each f As String In _
Directory.GetFiles(sourcePath)
Dim fInfo As New FileInfo(f)
Dim fileNode As New FileTreeNode(fInfo)
nodes.Add(fileNode)
Next
Catch ex As Exception
Console.WriteLine(ex.Message)
End Try
End Sub
Private Sub fileList_DoubleClick(ByVal sender As Object, _
ByVal e As System.EventArgs) _
Handles fileList.DoubleClick
If Not fileList.SelectedNode Is Nothing Then
Dim tn As TreeNode = fileList.SelectedNode
If TypeOf tn Is DirectoryTreeNode Then
Dim dtn As DirectoryTreeNode
dtn = DirectCast(tn, DirectoryTreeNode)
MsgBox("Directory Selected: " & dtn.fullName)
ElseIf TypeOf tn Is FileTreeNode Then
Dim ftn As FileTreeNode
ftn = DirectCast(tn, FileTreeNode)
MsgBox("File Selected. Created: " _
& ftn.creationTime)
End If
End If
End Sub
End Class
Public Class FileTreeNode
Inherits TreeNode
Protected m_creationTime As Date
Protected m_fullName As String
Protected m_lastAccessTime As Date
Protected m_lastWriteTime As Date
Protected m_length As Long
Public Sub New(ByVal fInfo As FileInfo)
With fInfo
m_creationTime = .CreationTime
m_fullName = .FullName
m_lastAccessTime = .LastAccessTime
m_lastWriteTime = .LastWriteTime
m_length = .Length
Text = .Name
End With
End Sub
Public Sub New()
End Sub
Public Property creationTime() As Date
Get
Return m_creationTime
End Get
Set(ByVal Value As Date)
m_creationTime = Value
End Set
End Property
Public Property fullName() As String
Get
Return m_fullName
End Get
Set(ByVal Value As String)
m_fullName = Value
End Set
End Property
Public Property lastAccessTime() As Date
Get
Return m_lastAccessTime
End Get
Set(ByVal Value As Date)
m_lastAccessTime = Value
End Set
End Property
Public Property lastWriteTime() As Date
Get
Return m_lastWriteTime
End Get
Set(ByVal Value As Date)
m_lastWriteTime = Value
End Set
End Property
Public Property length() As Long
Get
Return m_length
End Get
Set(ByVal Value As Long)
m_length = Value
End Set
End Property
End Class
Public Class DirectoryTreeNode
Inherits FileTreeNode
Public Sub New(ByVal dInfo As DirectoryInfo)
With dInfo
m_creationTime = .CreationTime
m_fullName = .FullName
m_lastAccessTime = .LastAccessTime
m_lastWriteTime = .LastWriteTime
m_length = 0
Text = .Name
End With
End Sub
End Class