<Window
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="GridLengthConverter_grid.Window1"
Title="GridLengthConverter Sample"
WindowState="Maximized">
<StackPanel>
<Grid Name="grid1" ShowGridLines="True" Height="300">
<Grid.ColumnDefinitions>
<ColumnDefinition Name="col1" />
<ColumnDefinition Name="col2" />
<ColumnDefinition Name="col3" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Name="row1" />
<RowDefinition Name="row2" />
<RowDefinition Name="row3" />
</Grid.RowDefinitions>
<TextBlock Grid.Row="0" Grid.Column="0" TextWrapping="Wrap">Column 0, Row 0</TextBlock>
<TextBlock Grid.Row="0" Grid.Column="1" TextWrapping="Wrap">Column 1, Row 0</TextBlock>
<TextBlock Grid.Row="0" Grid.Column="2" TextWrapping="Wrap">Column 2, Row 0</TextBlock>
<TextBlock Grid.Row="1" Grid.Column="0" TextWrapping="Wrap">Column 0, Row 1</TextBlock>
<TextBlock Grid.Row="1" Grid.Column="1" TextWrapping="Wrap">Column 1, Row 1</TextBlock>
<TextBlock Grid.Row="1" Grid.Column="2" TextWrapping="Wrap">Column 2, Row 1</TextBlock>
<TextBlock Grid.Row="2" Grid.Column="0" TextWrapping="Wrap">Column 0, Row 2</TextBlock>
<TextBlock Grid.Row="2" Grid.Column="1" TextWrapping="Wrap">Column 1, Row 2</TextBlock>
<TextBlock Grid.Row="2" Grid.Column="2" TextWrapping="Wrap">Column 2, Row 2</TextBlock>
</Grid>
<Slider Name="hs1" Width="100" IsSnapToTickEnabled="True" Maximum="2" TickPlacement="BottomRight" TickFrequency="1" ValueChanged="changeColVal"/>
<Slider Orientation="Horizontal" Name="hs2" Width="100" IsSnapToTickEnabled="True" Maximum="2" TickPlacement="BottomRight" TickFrequency="1" ValueChanged="changeRowVal" />
<TextBlock Name="txt1" TextWrapping="Wrap"/>
<TextBlock Name="txt2" TextWrapping="Wrap"/>
<ListBox Width="50" Height="50" SelectionChanged="changeCol">
<ListBoxItem>25</ListBoxItem>
<ListBoxItem>50</ListBoxItem>
<ListBoxItem>75</ListBoxItem>
<ListBoxItem>100</ListBoxItem>
<ListBoxItem>125</ListBoxItem>
<ListBoxItem>150</ListBoxItem>
</ListBox>
<ListBox Width="50" Height="50" SelectionChanged="changeRow">
<ListBoxItem>25</ListBoxItem>
<ListBoxItem>50</ListBoxItem>
<ListBoxItem>75</ListBoxItem>
<ListBoxItem>100</ListBoxItem>
<ListBoxItem>125</ListBoxItem>
<ListBoxItem>150</ListBoxItem>
</ListBox>
<Button Name="btn1" Click="setMinWidth">Set MinWidth="100"</Button>
<Button Name="btn2" Click="setMaxWidth">Set MaxWidth="125"</Button>
<Button Name="btn3" Click="setMinHeight">Set MinHeight="50"</Button>
<Button Name="btn4" Click="setMaxHeight">Set MaxHeight="75"</Button>
</StackPanel>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Documents
Namespace GridLengthConverter_grid
Public Partial Class Window1
Inherits Window
Public Sub changeRowVal(sender As Object, e As RoutedEventArgs)
txt2.Text = "Current Grid Row is " + hs2.Value.ToString()
End Sub
Public Sub changeColVal(sender As Object, e As RoutedEventArgs)
txt1.Text = "Current Grid Column is " + hs1.Value.ToString()
End Sub
Public Sub changeCol(sender As Object, args As SelectionChangedEventArgs)
Dim li As ListBoxItem = TryCast(TryCast(sender, ListBox).SelectedItem, ListBoxItem)
Dim myGridLengthConverter As New GridLengthConverter()
If hs1.Value = 0 Then
Dim gl1 As GridLength = CType(myGridLengthConverter.ConvertFromString(li.Content.ToString()), GridLength)
col1.Width = gl1
ElseIf hs1.Value = 1 Then
Dim gl2 As GridLength = CType(myGridLengthConverter.ConvertFromString(li.Content.ToString()), GridLength)
col2.Width = gl2
ElseIf hs1.Value = 2 Then
Dim gl3 As GridLength = CType(myGridLengthConverter.ConvertFromString(li.Content.ToString()), GridLength)
col3.Width = gl3
End If
End Sub
Public Sub changeRow(sender As Object, args As SelectionChangedEventArgs)
Dim li2 As ListBoxItem = TryCast(TryCast(sender, ListBox).SelectedItem, ListBoxItem)
Dim myGridLengthConverter2 As New GridLengthConverter()
If hs2.Value = 0 Then
Dim gl4 As GridLength = CType(myGridLengthConverter2.ConvertFromString(li2.Content.ToString()), GridLength)
row1.Height = gl4
ElseIf hs2.Value = 1 Then
Dim gl5 As GridLength = CType(myGridLengthConverter2.ConvertFromString(li2.Content.ToString()), GridLength)
row2.Height = gl5
ElseIf hs2.Value = 2 Then
Dim gl6 As GridLength = CType(myGridLengthConverter2.ConvertFromString(li2.Content.ToString()), GridLength)
row3.Height = gl6
End If
End Sub
Public Sub setMinWidth(sender As Object, e As RoutedEventArgs)
col1.MinWidth = 100
col2.MinWidth = 100
col3.MinWidth = 100
End Sub
Public Sub setMaxWidth(sender As Object, e As RoutedEventArgs)
col1.MaxWidth = 125
col2.MaxWidth = 125
col3.MaxWidth = 125
End Sub
Public Sub setMinHeight(sender As Object, e As RoutedEventArgs)
row1.MinHeight = 50
row2.MinHeight = 50
row3.MinHeight = 50
End Sub
Public Sub setMaxHeight(sender As Object, e As RoutedEventArgs)
row1.MaxHeight = 75
row2.MaxHeight = 75
row3.MaxHeight = 75
End Sub
End Class
End Namespace
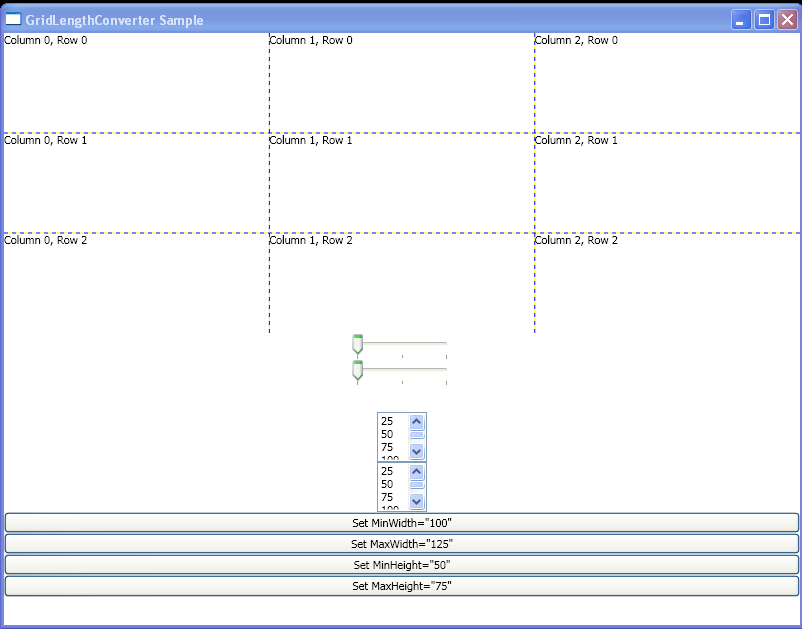