Creating Lines : Line « Windows Presentation Foundation « VB.Net Tutorial
- VB.Net Tutorial
- Windows Presentation Foundation
- Line
<Window x:Class="WpfApplication1.PerpendicularLine"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Perpendicular Line" Height="300" Width="400">
<Viewbox Stretch="Uniform">
<Canvas Name="canvas1" Grid.Column="1" Margin="10"
ClipToBounds="True" Width="270" Height="280">
<TextBlock Name="tbPoint1" Canvas.Top="10">
Point1
</TextBlock>
<TextBlock Name="tbPoint2" Canvas.Top="25">
Point2
</TextBlock>
<TextBlock Name="tbPoint3" Canvas.Top="40">
Point3
</TextBlock>
<TextBlock Name="tbPoint4" Canvas.Top="55">
Point4
</TextBlock>
</Canvas>
</Viewbox>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Media
Imports System.Windows.Shapes
Namespace WpfApplication1
Public Partial Class PerpendicularLine
Inherits Window
Private line1 As Line
Private line2 As Line
Public Sub New()
InitializeComponent()
Dim rect As New Rectangle()
rect.Stroke = Brushes.Black
rect.Width = canvas1.Width
rect.Height = canvas1.Height
canvas1.Children.Add(rect)
line1 = New Line()
line2 = New Line()
AddLines()
End Sub
Private Sub AddLines()
Dim pt1 As New Point()
Dim pt2 As New Point()
pt1.X = 30
pt1.Y = 300
pt2.X = 100
pt2.Y = 500
Dim length As Double = 400
line1 = New Line()
line1.X1 = pt1.X
line1.Y1 = pt1.Y
line1.X2 = pt2.X
line1.Y2 = pt2.Y
line1.Stroke = Brushes.Gray
line1.StrokeThickness = 4
canvas1.Children.Add(line1)
Canvas.SetLeft(tbPoint1, pt1.X)
Canvas.SetTop(tbPoint1, pt1.Y)
Canvas.SetLeft(tbPoint2, pt2.X)
Canvas.SetTop(tbPoint2, pt2.Y)
tbPoint1.Text = "Pt1(" & pt1.ToString() & ")"
tbPoint2.Text = "Pt2(" & pt2.ToString() & ")"
Dim v1 As Vector = pt1 - pt2
Dim m1 As New Matrix()
Dim pt3 As New Point()
Dim pt4 As New Point()
m1.Rotate(-90)
v1.Normalize()
v1 *= length
line2 = New Line()
line2.Stroke = Brushes.Gray
line2.StrokeThickness = 4
line2.StrokeDashArray = DoubleCollection.Parse("3, 1")
pt3 = pt2 + v1 * m1
m1 = New Matrix()
m1.Rotate(90)
pt4 = pt2 + v1 * m1
line2.X1 = pt3.X
line2.Y1 = pt3.Y
line2.X2 = pt4.X
line2.Y2 = pt4.Y
canvas1.Children.Add(line2)
Canvas.SetLeft(tbPoint3, pt3.X)
Canvas.SetTop(tbPoint3, pt3.Y)
Canvas.SetLeft(tbPoint4, pt4.X)
Canvas.SetTop(tbPoint4, pt4.Y)
pt3.X = Math.Round(pt3.X, 0)
pt3.Y = Math.Round(pt3.Y, 0)
pt4.X = Math.Round(pt4.X, 0)
pt4.Y = Math.Round(pt4.Y, 0)
tbPoint3.Text = "Pt3(" & pt3.ToString() & ")"
tbPoint4.Text = "Pt4(" & pt4.ToString() & ")"
End Sub
End Class
End Namespace
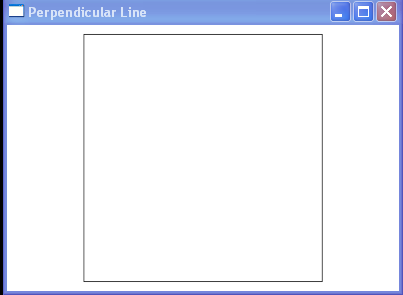