<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="" Height="300" Width="300"
>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Collections.Generic
Imports System.Text
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Data
Imports System.Windows.Documents
Imports System.Windows.Input
Imports System.Windows.Media
Imports System.Windows.Media.Imaging
Imports System.Windows.Shapes
Namespace WpfApplication1
Public Partial Class Window1
Inherits System.Windows.Window
Public Sub New()
InitializeComponent()
Dim g As New Grid()
Dim r As New RowDefinition()
r.Height = New GridLength(0, GridUnitType.Auto)
g.RowDefinitions.Add(r)
r = New RowDefinition()
r.Height = New GridLength(2, GridUnitType.Star)
g.RowDefinitions.Add(r)
r = New RowDefinition()
r.Height = New GridLength(1, GridUnitType.Star)
g.RowDefinitions.Add(r)
Dim c As New ColumnDefinition()
c.Width = New GridLength(0, GridUnitType.Auto)
g.ColumnDefinitions.Add(c)
c = New ColumnDefinition()
g.ColumnDefinitions.Add(c)
Dim tb As New TextBlock()
tb.Text = "Protocol:"
Grid.SetColumn(tb, 0)
Grid.SetRow(tb, 0)
g.Children.Add(tb)
tb = New TextBlock()
tb.Text = "HyperText Transfer Protocol:"
Grid.SetColumn(tb, 1)
Grid.SetRow(tb, 0)
g.Children.Add(tb)
tb = New TextBlock()
tb.Text = "Type:"
Grid.SetColumn(tb, 0)
Grid.SetRow(tb, 1)
g.Children.Add(tb)
tb = New TextBlock()
tb.Text = "HTML Document"
Grid.SetColumn(tb, 1)
Grid.SetRow(tb, 1)
g.Children.Add(tb)
tb = New TextBlock()
tb.Text = "Connection:"
Grid.SetColumn(tb, 0)
Grid.SetRow(tb, 2)
g.Children.Add(tb)
tb = New TextBlock()
tb.Text = "Not encrypted"
Grid.SetColumn(tb, 1)
Grid.SetRow(tb, 2)
g.Children.Add(tb)
g.ShowGridLines = True
Me.Content = g
End Sub
End Class
End Namespace
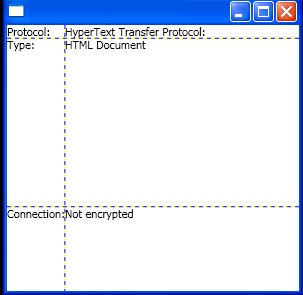