<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="LogicalVisualTreeSample" Height="300" Width="300">
<WrapPanel Name="rootPanel">
<Button>_Click me</Button>
</WrapPanel>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Collections.Generic
Imports System.Text
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Data
Imports System.Windows.Documents
Imports System.Windows.Input
Imports System.Windows.Media
Imports System.Windows.Media.Imaging
Imports System.Windows.Shapes
Imports System.Diagnostics
Namespace WpfApplication1
Public Partial Class Window1
Inherits System.Windows.Window
Public Sub New()
InitializeComponent()
DumpLogicalTree(rootPanel)
End Sub
Protected Overrides Sub OnContentRendered(e As EventArgs)
MyBase.OnContentRendered(e)
DumpVisualTree(rootPanel)
End Sub
Private Sub DumpLogicalTree(parent As Object)
Dim typeName As String = parent.[GetType]().Name
Dim name As String = Nothing
Dim doParent As DependencyObject = TryCast(parent, DependencyObject)
If doParent IsNot Nothing Then
name = DirectCast(If(doParent.GetValue(FrameworkElement.NameProperty), ""), String)
Else
name = parent.ToString()
End If
Debug.WriteLine(typeName)
Console.WriteLine(name)
If doParent Is Nothing Then
Return
End If
For Each child As Object In LogicalTreeHelper.GetChildren(doParent)
DumpLogicalTree(child)
Next
End Sub
Private Sub DumpVisualTree(parent As DependencyObject)
Dim typeName As String = parent.[GetType]().Name
Dim name As String = DirectCast(If(parent.GetValue(FrameworkElement.NameProperty), ""), String)
Debug.WriteLine(typeName)
Console.WriteLine(name)
Dim i As Integer = 0
While i <> VisualTreeHelper.GetChildrenCount(parent)
Dim child As DependencyObject = VisualTreeHelper.GetChild(parent, i)
DumpVisualTree(child)
i += 1
End While
End Sub
End Class
End Namespace
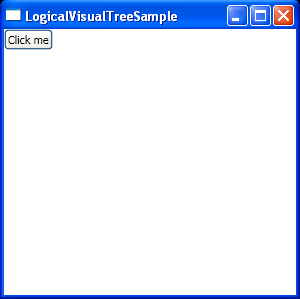