<Window
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="WpfApplication1.Window1"
Title="Color Spinner" Height="370" Width="270" >
<Window.Resources>
<Storyboard x:Key="Spin">
<DoubleAnimationUsingKeyFrames BeginTime="00:00:00" Storyboard.TargetName="ellipse1" Storyboard.TargetProperty="(UIElement.RenderTransform).(TransformGroup.Children)[0].(RotateTransform.Angle)" RepeatBehavior="Forever">
<SplineDoubleKeyFrame KeyTime="00:00:10" Value="360"/>
</DoubleAnimationUsingKeyFrames>
</Storyboard>
</Window.Resources>
<Window.Triggers>
<EventTrigger RoutedEvent="FrameworkElement.Loaded">
<BeginStoryboard Storyboard="{StaticResource Spin}" x:Name="Spin_BeginStoryboard"/>
</EventTrigger>
<EventTrigger RoutedEvent="ButtonBase.Click" SourceName="goButton">
<ResumeStoryboard BeginStoryboardName="Spin_BeginStoryboard"/>
</EventTrigger>
<EventTrigger RoutedEvent="ButtonBase.Click" SourceName="stopButton">
<PauseStoryboard BeginStoryboardName="Spin_BeginStoryboard"/>
</EventTrigger>
</Window.Triggers>
<Window.Background>
<LinearGradientBrush EndPoint="0.5,1" StartPoint="0.5,0">
<GradientStop Color="#FFFFFFFF" Offset="0"/>
<GradientStop Color="#FFFFC45A" Offset="1"/>
</LinearGradientBrush>
</Window.Background>
<Grid>
<StackPanel>
<Ellipse Margin="15,85,0,0" Name="ellipse1" Stroke="{x:Null}" Height="80" HorizontalAlignment="Left" VerticalAlignment="Top" Width="120" Fill="Red" Opacity="0.5" RenderTransformOrigin="0.92,0.5" >
<Ellipse.BitmapEffect>
<BevelBitmapEffect/>
</Ellipse.BitmapEffect>
<Ellipse.RenderTransform>
<TransformGroup>
<RotateTransform Angle="0"/>
</TransformGroup>
</Ellipse.RenderTransform>
</Ellipse>
<Button Name="goButton" Content="Go"/>
<Button Name="stopButton" Content="Stop"/>
<Button Name="toggleButton" Content="Toggle" Click="toggleButton_Click" />
</StackPanel>
</Grid>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Text
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Data
Imports System.Windows.Documents
Imports System.Windows.Input
Imports System.Windows.Media
Imports System.Windows.Media.Imaging
Imports System.Windows.Navigation
Imports System.Windows.Shapes
Imports System.Windows.Media.Animation
Namespace WpfApplication1
Public Partial Class Window1
Inherits Window
Public Sub New()
InitializeComponent()
End Sub
Private Sub toggleButton_Click(sender As Object, e As RoutedEventArgs)
Dim spinStoryboard As Storyboard = TryCast(Resources("Spin"), Storyboard)
If spinStoryboard IsNot Nothing Then
If spinStoryboard.GetIsPaused(Me) Then
spinStoryboard.[Resume](Me)
Else
spinStoryboard.Pause(Me)
End If
End If
End Sub
End Class
End Namespace
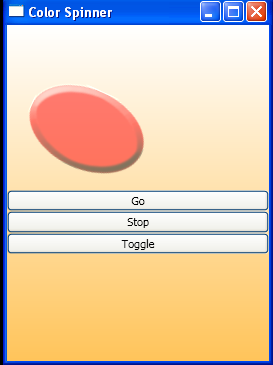