<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" Height="100" Width="200">
<StackPanel>
<ProgressBar Name="progressBar" Margin="4"/>
<Button Name="button" Click="button_Click">Start</Button>
</StackPanel>
</Window>
//File:Window.xaml.vb
Imports System.ComponentModel
Imports System.Threading
Imports System.Windows
Imports System.Windows.Input
Namespace WpfApplication1
Public Partial Class Window1
Inherits Window
Private worker As New BackgroundWorker()
Public Sub New()
InitializeComponent()
worker.WorkerReportsProgress = True
AddHandler worker.DoWork, New DoWorkEventHandler(AddressOf worker_DoWork)
AddHandler worker.RunWorkerCompleted, New RunWorkerCompletedEventHandler(AddressOf worker_RunWorkerCompleted)
AddHandler worker.ProgressChanged, AddressOf worker_ProgressChanged
End Sub
Private Sub button_Click(sender As Object, e As RoutedEventArgs)
worker.RunWorkerAsync()
Me.Cursor = Cursors.Wait
button.IsEnabled = False
End Sub
Private Sub worker_RunWorkerCompleted(sender As Object, e As RunWorkerCompletedEventArgs)
Me.Cursor = Cursors.Arrow
If e.[Error] IsNot Nothing Then
MessageBox.Show(e.[Error].Message)
End If
button.IsEnabled = True
End Sub
Private Sub worker_DoWork(sender As Object, e As DoWorkEventArgs)
For i As Integer = 1 To 100
Thread.Sleep(100)
worker.ReportProgress(i)
Next
End Sub
Private Sub worker_ProgressChanged(sender As Object, e As ProgressChangedEventArgs)
progressBar.Value = e.ProgressPercentage
End Sub
End Class
End Namespace
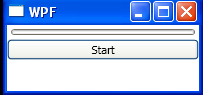