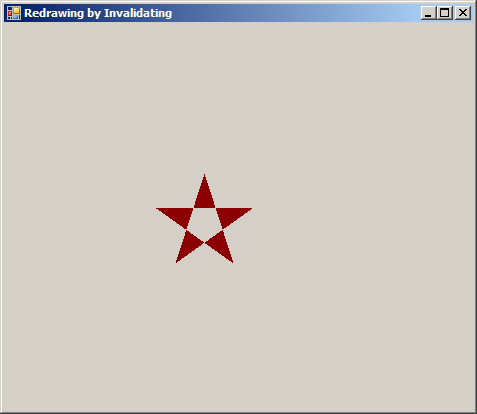
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Windows.Forms
public class DrawStart5Alternate
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Private Sub Form1_Paint(ByVal sender As Object, _
ByVal e As System.Windows.Forms.PaintEventArgs) _
Handles Me.Paint
Dim canvas As Graphics = e.Graphics
Dim starPoints(4) As Point
Dim angle As Double
Dim radians As Double
Dim pointX As Double
Dim pointY As Double
Dim counter As Integer
Const pointDistance As Double = 50
Const angleStart As Integer = 198
Const angleRotation As Integer = 144
angle = angleStart
For counter = 0 To 4
angle += angleRotation
radians = angle * Math.PI / 180
pointX = Math.Cos(radians) * pointDistance +200
pointY = Math.Sin(radians) * pointDistance +200
starPoints(counter) = New Point(CInt(pointX), CInt(pointY))
Next counter
canvas.FillPolygon(Brushes.DarkRed, starPoints, Drawing2D.FillMode.Alternate)
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.SuspendLayout()
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(469, 387)
Me.Name = "Form1"
Me.Text = "Redrawing by Invalidating"
Me.ResumeLayout(False)
End Sub
End Class