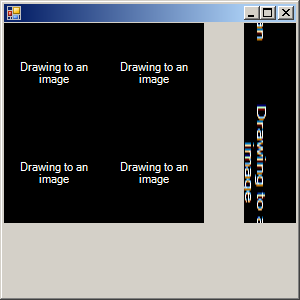
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Windows.Forms
public class TransformTextureBrush
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
public class Form1
Inherits System.Windows.Forms.Form
Protected Overrides Sub OnPaint(ByVal e As PaintEventArgs)
Dim g As Graphics = Me.CreateGraphics()
g.Clear(Me.BackColor)
' Create a TextureBrush object
Dim txtrBrush As New TextureBrush(New Bitmap("yourfile.jpg"))
' Create a transformation matrix.
Dim M As New Matrix
' Rotate the texture image by 90 degrees.
txtrBrush.RotateTransform(90, MatrixOrder.Prepend)
' Translate
M.Translate(50, 0)
' Multiply the transformation matrix
' of tBrush by translateMatrix.
txtrBrush.MultiplyTransform(M)
' Scale operation
txtrBrush.ScaleTransform(2, 1, MatrixOrder.Prepend)
' Fill a rectangle with texture brush
g.FillRectangle(txtrBrush, 240, 0, 200, 200)
' Reset transformation
txtrBrush.ResetTransform()
' Fill rectangle after reseting transformation
g.FillRectangle(txtrBrush, 0, 0, 200, 200)
' Dispose
txtrBrush.Dispose()
g.Dispose()
End Sub
Public Sub New()
MyBase.New()
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen
End Sub
End Class