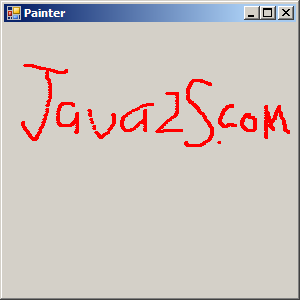
Imports System.Windows.Forms
Imports System.Drawing
Imports System.Drawing.Drawing2D
public class MouseDrawForm
public Shared Sub Main
Application.Run(New FrmPainter)
End Sub
End class
Public Class FrmPainter
Inherits System.Windows.Forms.Form
Dim shouldPaint As Boolean = False
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Name = "Form1"
Me.Text = "Painter"
End Sub
#End Region
Private Sub FrmPainter_MouseMove( _
ByVal sender As System.Object, _
ByVal e As System.Windows.Forms.MouseEventArgs) _
Handles MyBase.MouseMove
If shouldPaint Then
Dim graphic As Graphics = CreateGraphics()
graphic.FillEllipse(New SolidBrush(Color.Red), e.X, e.Y, 4, 4)
End If
End Sub
Private Sub FrmPainter_MouseDown(ByVal sender As Object, _
ByVal e As System.Windows.Forms.MouseEventArgs) _
Handles MyBase.MouseDown
shouldPaint = True
End Sub
Private Sub FrmPainter_MouseUp(ByVal sender As Object, _
ByVal e As System.Windows.Forms.MouseEventArgs) _
Handles MyBase.MouseUp
shouldPaint = False
End Sub
End Class