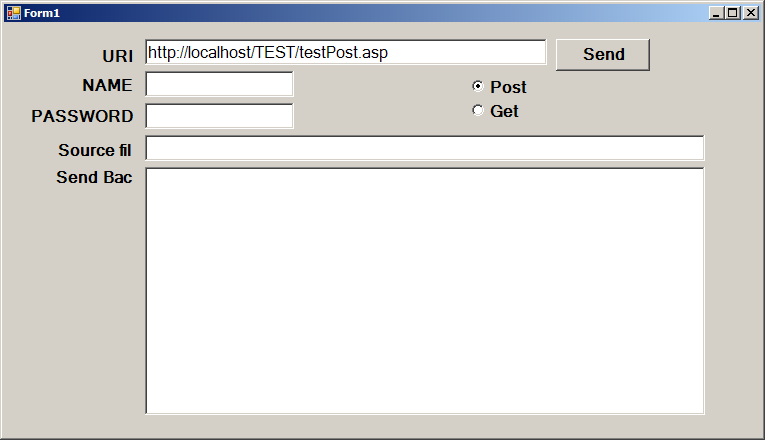
Imports System
Imports System.IO
Imports System.Net
Imports System.Web
Imports System.Text
Imports System.Windows.Forms
public class ReadAspPage
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Inherits System.Windows.Forms.Form
Public Sub New()
MyBase.New()
InitializeComponent()
End Sub
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Private components As System.ComponentModel.IContainer
Friend WithEvents btnSend As System.Windows.Forms.Button
Friend WithEvents lblURI As System.Windows.Forms.Label
Friend WithEvents txtURI As System.Windows.Forms.TextBox
Friend WithEvents txtContent As System.Windows.Forms.TextBox
Friend WithEvents rbGet As System.Windows.Forms.RadioButton
Friend WithEvents rbPost As System.Windows.Forms.RadioButton
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents Label2 As System.Windows.Forms.Label
Friend WithEvents txtName As System.Windows.Forms.TextBox
Friend WithEvents Label3 As System.Windows.Forms.Label
Friend WithEvents txtPassword As System.Windows.Forms.TextBox
Friend WithEvents Label4 As System.Windows.Forms.Label
Friend WithEvents txtSourceUri As System.Windows.Forms.TextBox
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.btnSend = New System.Windows.Forms.Button()
Me.lblURI = New System.Windows.Forms.Label()
Me.txtURI = New System.Windows.Forms.TextBox()
Me.txtContent = New System.Windows.Forms.TextBox()
Me.rbGet = New System.Windows.Forms.RadioButton()
Me.rbPost = New System.Windows.Forms.RadioButton()
Me.Label1 = New System.Windows.Forms.Label()
Me.Label2 = New System.Windows.Forms.Label()
Me.txtName = New System.Windows.Forms.TextBox()
Me.Label3 = New System.Windows.Forms.Label()
Me.txtPassword = New System.Windows.Forms.TextBox()
Me.Label4 = New System.Windows.Forms.Label()
Me.txtSourceUri = New System.Windows.Forms.TextBox()
Me.SuspendLayout()
'
'btnSend
'
Me.btnSend.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.btnSend.Location = New System.Drawing.Point(604, 16)
Me.btnSend.Name = "btnSend"
Me.btnSend.Size = New System.Drawing.Size(103, 32)
Me.btnSend.TabIndex = 12
Me.btnSend.Text = "Send"
'
'lblURI
'
Me.lblURI.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.lblURI.Location = New System.Drawing.Point(92, 23)
Me.lblURI.Name = "lblURI"
Me.lblURI.Size = New System.Drawing.Size(51, 16)
Me.lblURI.TabIndex = 11
Me.lblURI.Text = "URI"
Me.lblURI.TextAlign = System.Drawing.ContentAlignment.TopRight
'
'txtURI
'
Me.txtURI.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.txtURI.Location = New System.Drawing.Point(154, 16)
Me.txtURI.Name = "txtURI"
Me.txtURI.Size = New System.Drawing.Size(440, 26)
Me.txtURI.TabIndex = 10
Me.txtURI.Text = "http://localhost/TEST/testPost.asp"
'
'txtContent
'
Me.txtContent.Location = New System.Drawing.Point(154, 144)
Me.txtContent.Multiline = True
Me.txtContent.Name = "txtContent"
Me.txtContent.Size = New System.Drawing.Size(614, 248)
Me.txtContent.TabIndex = 13
Me.txtContent.Text = ""
'
'rbGet
'
Me.rbGet.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.rbGet.Location = New System.Drawing.Point(512, 80)
Me.rbGet.Name = "rbGet"
Me.rbGet.Size = New System.Drawing.Size(82, 16)
Me.rbGet.TabIndex = 14
Me.rbGet.Text = "Get"
'
'rbPost
'
Me.rbPost.Checked = True
Me.rbPost.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.rbPost.Location = New System.Drawing.Point(512, 56)
Me.rbPost.Name = "rbPost"
Me.rbPost.Size = New System.Drawing.Size(82, 16)
Me.rbPost.TabIndex = 15
Me.rbPost.TabStop = True
Me.rbPost.Text = "Post"
'
'Label1
'
Me.Label1.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.Label1.Location = New System.Drawing.Point(51, 144)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(92, 16)
Me.Label1.TabIndex = 16
Me.Label1.Text = "Send Back"
Me.Label1.TextAlign = System.Drawing.ContentAlignment.TopRight
'
'Label2
'
Me.Label2.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.Label2.Location = New System.Drawing.Point(72, 52)
Me.Label2.Name = "Label2"
Me.Label2.Size = New System.Drawing.Size(71, 16)
Me.Label2.TabIndex = 18
Me.Label2.Text = "NAME"
Me.Label2.TextAlign = System.Drawing.ContentAlignment.TopRight
'
'txtName
'
Me.txtName.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.txtName.Location = New System.Drawing.Point(154, 48)
Me.txtName.Name = "txtName"
Me.txtName.Size = New System.Drawing.Size(163, 26)
Me.txtName.TabIndex = 17
Me.txtName.Text = ""
'
'Label3
'
Me.Label3.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.Label3.Location = New System.Drawing.Point(20, 83)
Me.Label3.Name = "Label3"
Me.Label3.Size = New System.Drawing.Size(123, 16)
Me.Label3.TabIndex = 20
Me.Label3.Text = "PASSWORD"
Me.Label3.TextAlign = System.Drawing.ContentAlignment.TopRight
'
'txtPassword
'
Me.txtPassword.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.txtPassword.Location = New System.Drawing.Point(154, 80)
Me.txtPassword.Name = "txtPassword"
Me.txtPassword.Size = New System.Drawing.Size(163, 26)
Me.txtPassword.TabIndex = 19
Me.txtPassword.Text = ""
'
'Label4
'
Me.Label4.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.Label4.Location = New System.Drawing.Point(51, 117)
Me.Label4.Name = "Label4"
Me.Label4.Size = New System.Drawing.Size(92, 16)
Me.Label4.TabIndex = 22
Me.Label4.Text = "Source file"
Me.Label4.TextAlign = System.Drawing.ContentAlignment.TopRight
'
'txtSourceUri
'
Me.txtSourceUri.Font = New System.Drawing.Font("Microsoft Sans Serif", 12.0!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(136, Byte))
Me.txtSourceUri.Location = New System.Drawing.Point(154, 112)
Me.txtSourceUri.Name = "txtSourceUri"
Me.txtSourceUri.Size = New System.Drawing.Size(614, 26)
Me.txtSourceUri.TabIndex = 21
Me.txtSourceUri.Text = ""
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(6, 14)
Me.ClientSize = New System.Drawing.Size(829, 413)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.Label4, Me.txtSourceUri, Me.Label3, Me.txtPassword, Me.Label2, Me.txtName, Me.Label1, Me.rbPost, Me.rbGet, Me.txtContent, Me.btnSend, Me.lblURI, Me.txtURI})
Me.Name = "Form1"
Me.Text = "Form1"
Me.ResumeLayout(False)
End Sub
Private Sub btnSend_Click _
(ByVal sender As System.Object, ByVal e As System.EventArgs) _
Handles btnSend.Click
Select Case rbPost.Checked
Case True
PostData()
Case False
GetData()
End Select
End Sub
Private Sub PostData()
Dim strURL As String = txtURI.Text
Dim strPost As String
Dim myHttpWebRequest As HttpWebRequest = _
CType(WebRequest.Create(strURL), HttpWebRequest)
strPost = "NAME=" & txtName.Text & "&" & "PASS=" & txtPassword.Text
SetWRProperty(myHttpWebRequest, "POST", strPost.Length, "application/x-www-form-urlencoded")
WriteData(myHttpWebRequest, strPost)
Dim myHttpWebResponse As HttpWebResponse = CType(myHttpWebRequest.GetResponse(), HttpWebResponse)
GetResponseData(myHttpWebResponse.GetResponseStream())
txtSourceUri.Text = myHttpWebRequest.RequestUri.ToString
myHttpWebResponse.Close()
End Sub
Private Sub GetData()
Dim strURL As String = txtURI.Text
Dim myStreamWriter As StreamWriter = Nothing
Dim strGET As String
Dim myHttpWebRequest As HttpWebRequest
strGET = "NAME=" & txtName.Text & "&" & "PASS=" & txtPassword.Text
myHttpWebRequest = CType(WebRequest.Create(strURL + "?" + strGET), HttpWebRequest)
Dim myHttpWebResponse As HttpWebResponse = _
CType(myHttpWebRequest.GetResponse(), HttpWebResponse)
GetResponseData(myHttpWebResponse.GetResponseStream())
txtSourceUri.Text = myHttpWebRequest.RequestUri.ToString
myHttpWebResponse.Close()
End Sub
Private Sub GetResponseData(ByVal myStream As Stream)
Dim myStreamReader As StreamReader = New StreamReader(myStream)
Dim strOut As String = myStreamReader.ReadToEnd()
txtContent.Text = strOut
myStreamReader.Close()
End Sub
Private Sub SetWRProperty(ByRef pHttpWR As HttpWebRequest, _
ByVal pMethod As String, ByVal pLength As Long, ByVal strType As String)
pHttpWR.Method = pMethod
pHttpWR.ContentLength = pLength
pHttpWR.ContentType = strType
End Sub
Private Sub WriteData(ByRef pHttpWR As HttpWebRequest, ByVal pstrPost As String)
Dim myStreamWriter As StreamWriter = Nothing
myStreamWriter = New StreamWriter(pHttpWR.GetRequestStream())
myStreamWriter.Write(pstrPost)
myStreamWriter.Close()
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
End Sub
End Class
'testGet.asp
'<html>
'<head>
'<meta http-equiv="Content-Type" content="text/html; charset=big5">
'<meta name="GENERATOR" content="Microsoft FrontPage 4.0">
'<meta name="ProgId" content="FrontPage.Editor.Document">
'</head>
'<body>
'NAMEG<%response.write(REQUEST.QUERYSTRING("NAME"))%>
'<BR>
'PASSWORDG<%response.write(REQUEST.QUERYSTRING("PASS"))%>
'</p>
'<form method="GET" action="testGET.asp">
' <P>NAME<input type="text" name="NAME" size="20"></p>
' <P>PASSWORD<input type="text" name="PASS" size="20"></p>
' <input type="submit" value="submit" name="B1">
'</form>
'</body>
'</html>
'''''''''''''''''''''''''''''''''''''''''''''''''''
'testPost
'<html>
'<head>
'<meta http-equiv="Content-Type" content="text/html; charset=big5">
'<meta name="GENERATOR" content="Microsoft FrontPage 4.0">
'<meta name="ProgId" content="FrontPage.Editor.Document">
'</head>
'<body>
'NAMEG<%response.write(REQUEST.Form("NAME"))%>
'<br>
'PASSWORDG<%response.write(REQUEST.Form("PASS"))%>
'</p>
'<form method="POST" action="testpost.asp">
' <P>NAME<input type="text" name="NAME" size="20"></p>
' <P>PASSWORD<input type="text" name="PASS" size="20"></p>
' <input type="submit" value="submit" name="B1">
'</form>
'</body>
'</html>
22.30.ASP Post |
| 22.30.1. | Post to Asp page | 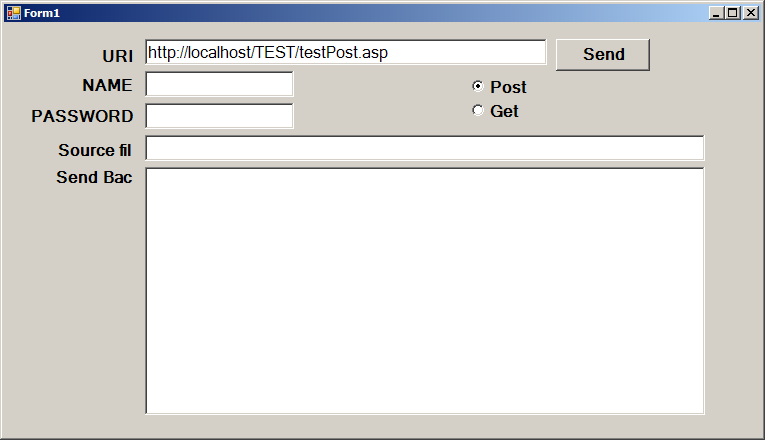 |