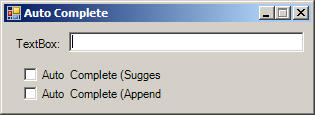
Imports System.Runtime.InteropServices
Imports System.Windows.Forms
public class AutoComplete
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Inherits System.Windows.Forms.Form
Public Sub New()
MyBase.New()
InitializeComponent()
End Sub
Private components As System.ComponentModel.IContainer
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents TextBox1 As System.Windows.Forms.TextBox
Friend WithEvents CheckBox1 As System.Windows.Forms.CheckBox
Friend WithEvents CheckBox2 As System.Windows.Forms.CheckBox
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.Label1 = New System.Windows.Forms.Label()
Me.TextBox1 = New System.Windows.Forms.TextBox()
Me.CheckBox1 = New System.Windows.Forms.CheckBox()
Me.CheckBox2 = New System.Windows.Forms.CheckBox()
Me.SuspendLayout()
'
'Label1
'
Me.Label1.Location = New System.Drawing.Point(10, 15)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(70, 20)
Me.Label1.TabIndex = 0
Me.Label1.Text = "TextBox:"
'
'TextBox1
'
Me.TextBox1.Location = New System.Drawing.Point(65, 10)
Me.TextBox1.Name = "TextBox1"
Me.TextBox1.Size = New System.Drawing.Size(235, 22)
Me.TextBox1.TabIndex = 1
Me.TextBox1.Text = ""
'
'CheckBox1
'
Me.CheckBox1.Location = New System.Drawing.Point(20, 45)
Me.CheckBox1.Name = "CheckBox1"
Me.CheckBox1.Size = New System.Drawing.Size(145, 20)
Me.CheckBox1.TabIndex = 2
Me.CheckBox1.Text = "Auto Complete (Suggest)"
'
'CheckBox2
'
Me.CheckBox2.Location = New System.Drawing.Point(20, 65)
Me.CheckBox2.Name = "CheckBox2"
Me.CheckBox2.Size = New System.Drawing.Size(145, 20)
Me.CheckBox2.TabIndex = 3
Me.CheckBox2.Text = "Auto Complete (Append)"
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 15)
Me.ClientSize = New System.Drawing.Size(307, 93)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.CheckBox2, Me.CheckBox1, Me.TextBox1, Me.Label1})
Me.MaximizeBox = False
Me.Name = "Form1"
Me.Text = "Auto Complete"
Me.ResumeLayout(False)
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
AutoComplete()
End Sub
Private Sub CheckBox1_CheckStateChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles CheckBox1.CheckStateChanged
AutoComplete()
End Sub
Private Sub CheckBox2_CheckStateChanged(ByVal sender As Object, ByVal e As System.EventArgs) Handles CheckBox2.CheckStateChanged
AutoComplete()
End Sub
Private Sub AutoComplete()
Dim dwFlags As Integer
If CheckBox1.CheckState Then
dwFlags = SHACF_URLALL Or SHACF_FILESYSTEM Or SHACF_AUTOSUGGEST_FORCE_ON
If CheckBox2.CheckState Then
dwFlags = dwFlags Or SHACF_AUTOAPPEND_FORCE_ON
Else
dwFlags = dwFlags Or SHACF_AUTOAPPEND_FORCE_OFF
End If
Else
dwFlags = SHACF_URLALL Or SHACF_FILESYSTEM Or SHACF_AUTOSUGGEST_FORCE_OFF
If CheckBox2.CheckState Then
dwFlags = dwFlags Or SHACF_AUTOAPPEND_FORCE_ON
Else
dwFlags = dwFlags Or SHACF_AUTOAPPEND_FORCE_OFF
End If
End If
SHAutoComplete(TextBox1.Handle.ToInt32, dwFlags)
End Sub
Public Const SHACF_DEFAULT As Integer = &H0S
Public Const SHACF_FILESYSTEM As Integer = &H1S
Public Const SHACF_URLHISTORY As Integer = &H2S
Public Const SHACF_URLMRU As Integer = &H4S
Public Const SHACF_USETAB As Integer = &H8S
Public Const SHACF_FILESYS_ONLY As Integer = &H10S
Public Const SHACF_AUTOSUGGEST_FORCE_ON As Integer = &H10000000
Public Const SHACF_AUTOSUGGEST_FORCE_OFF As Integer = &H20000000
Public Const SHACF_AUTOAPPEND_FORCE_ON As Integer = &H40000000
Public Const SHACF_AUTOAPPEND_FORCE_OFF As Integer = &H80000000
Public Const SHACF_URLALL As Integer = (SHACF_URLHISTORY Or SHACF_URLMRU)
Public Declare Function SHAutoComplete Lib "Shlwapi.dll" (ByVal hwndEdit As Integer, ByVal dwFlags As Integer) As Integer
End Class
24.15.IE Address Book |
| 24.15.1. | Address box autocomplete | 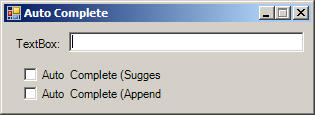 |
| 24.15.2. | Open URL | 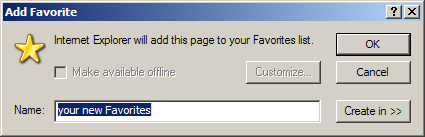 |