Javascript Date
Javascript Date type stores dates as the number of milliseconds that have passed since midnight on
January 1, 1970 UTC (Universal Time Code).
Create Current Date
To create a date object representing the current date and time:
var now = new Date();
console.log(now);
The code above generates the following result.

Create Date From millisecond
To create a date from the millisecond passed after midnight, January 1, 1970 UTC.
var aDate = new Date(1234567890);
console.log(aDate);
The code above generates the following result.

Create Date From string
For instance, to create a date object for May 25, 2004, you can use the following code:
var someDate = new Date("May 25, 2004");
console.log(someDate);
If the string doesn't represent a date, then it returns NaN.
The code above generates the following result.

Create Date From now
var start = Date.now();
console.log(start);
The code above generates the following result.

Create Date From parts
We can pass in the following date part to constructor to create Date object
- year,
- zero-based month (January is 0, February is 1, and so on),
- day of the month (1 through 31),
- hours (0 through 23)
- minutes
- seconds
- milliseconds
Of these arguments, only year and month are required.
If the day of the month isn't supplied, it's assumed
to be 1, while all other omitted arguments are assumed to be 0.
//January 1, 2000 at midnight in local time
var y2k = new Date(2000, 0);
console.log(y2k);
//May 5, 2005 at 5:55:55 PM local time
var aDate = new Date(2005, 4, 5, 17, 55, 55);
console.log(aDate);
The code above generates the following result.

Date Time Component Methods
The following methods of the Date type deal directly with getting and setting specific parts of the date value.
- getTime() - Returns the milliseconds representation of the date; same as valueOf().
- setTime(milliseconds) - Sets time in the milliseconds.
- getFullYear() - Returns the four-digit year.
- getUTCFullYear() - Returns the four-digit year of the UTC date value.
- setFullYear(year) - Sets the year with four digits to the date.
- setUTCFullYear(year) - Sets the yea with fourdigitsr of the UTC date.
- getMonth() - Returns the month of the date, where 0 represents January and 11 represents December.
- getUTCMonth() - Returns the month of the UTC date, where 0 represents January and 11 represents December.
- setMonth(month) - Sets the month of the date, which is any number 0 or greater. Numbers greater than 11 add years.
- setUTCMonth(month) - Sets the month of the UTC date, which is any number 0 or greater. Numbers greater than 11 add years.
- getDate() - Returns the day of the month (1 through 31) for the date.
- getUTCDate() - Returns the day of the month (1 through 31) for the UTC date.
- setDate(date) - Sets the day of the month for the date. If the date is greater than the number of days in the month, the month value gets increased.
- setUTCDate(date) - Sets the day of the month for the UTC date. If the date is greater than the number of days in the month, the month value gets increased.
- getDay() - Returns the date's day of the week as a number (where 0 represents Sunday and 6 represents Saturday).
- getUTCDay() - Returns the UTC date's day of the week as a number (where 0 represents Sunday and 6 represents Saturday).
- getHours() - Returns the date's hours as a number between 0 and 23.
- getUTCHours() - Returns the UTC date's hours as a number between 0 and 23.
- setHours (hours) - Sets the date's hours. A number greater than 23 increments the day of the month.
- setUTCHours(hours) - Sets the UTC date's hours. A number greater than 23 increments the day of the month.
- getMinutes() - Returns the date's minutes as a number between 0 and 59.
- getUTCMinutes() - Returns the UTC date's minutes as a number between 0 and 59.
- setMinutes(minutes) - Sets the date's minutes. A number greater than 59 increments the hour.
- setUTCMinutes(minutes) - Sets the UTC date's minutes. A number greater than 59 also increments the hour.
- getSeconds() - Returns the date's seconds as a number between 0 and 59.
- getUTCSeconds() - Returns the UTC date's seconds as a number between 0 and 59.
- setSeconds(seconds) - Sets the date's seconds. A number greater than 59 also increments the minutes.
- setUTCSeconds(seconds) - Sets the UTC date's seconds. Value greater than 59 increments the minutes.
- getMilliseconds() - Returns the date's milliseconds.
- getUTCMilliseconds() - Returns the UTC date's milliseconds.
- setMilliseconds(milliseconds) - Sets the date's milliseconds.
- setUTCMilliseconds(milliseconds) - Sets the UTC date's milliseconds.
- getTimezoneOffset() - Returns the number of minutes that the local time zone is off set from UTC.
var myDate = new Date();
myDate.setUTCFullYear(2012);/*from w w w . j av a 2s. c o m*/
myDate.setFullYear(2012);
myDate.setUTCSeconds(12);
myDate.setUTCMinutes(21);
myDate.setMilliseconds(123);
myDate.setUTCMilliseconds(12);
myDate.setUTCHours(1);
myDate.setUTCDate(23);
myDate.setHours(3);
myDate.setMinutes(12);
myDate.setSeconds(21);
myDate.setUTCMonth(01);
myDate.setMonth(2);//the month value starting from 0
myDate.setTime(123);
myDate.setDate(12);
console.log(myDate);
console.log(myDate.getUTCHours());
console.log(myDate.getUTCMonth());
console.log(myDate.getMinutes());
console.log(myDate.getUTCFullYear());
console.log(myDate.getFullYear());
console.log(myDate.getHours());
console.log(myDate.getDay());
console.log(myDate.getMilliseconds());
console.log(myDate.getUTCDate());
console.log(myDate.getDate());
console.log(myDate.getSeconds());
console.log(myDate.getMonth());
console.log(myDate.getTime());
console.log(myDate.getUTCDay());
console.log(myDate.getTimezoneOffset());
The code above generates the following result.
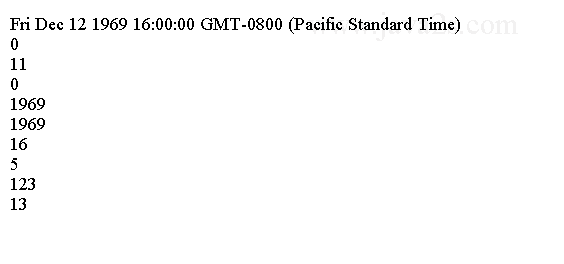