Get Image Resolution and Image size and Display size
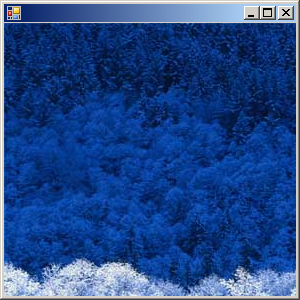
using System;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Drawing.Imaging;
public class Form1 : System.Windows.Forms.Form
{
public Form1()
{
InitializeComponent();
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Text = "";
this.Resize += new System.EventHandler(this.Form1_Resize);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.Form1_Paint);
}
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
Bitmap bmp = new Bitmap("winter.jpg");
g.DrawImage(bmp, 0, 0);
Console.WriteLine("Screen resolution: " + g.DpiX + "DPI");
Console.WriteLine("Image resolution: " + bmp.HorizontalResolution + "DPI");
Console.WriteLine("Image Width: " + bmp.Width);
Console.WriteLine("Image Height: " + bmp.Height);
SizeF s = new SizeF(bmp.Width * (g.DpiX / bmp.HorizontalResolution),
bmp.Height * (g.DpiY / bmp.VerticalResolution));
Console.WriteLine("Display size of image: " + s);
}
private void Form1_Resize(object sender, System.EventArgs e)
{
Invalidate();
}
}
Related examples in the same category