Add exception handling to the queue classes
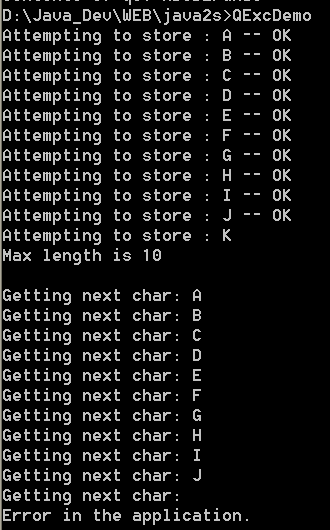
/*
C# A Beginner's Guide
By Schildt
Publisher: Osborne McGraw-Hill
ISBN: 0072133295
*/
/*
Project 10-1
Add exception handling to the queue classes.
*/
using System;
// A character queue interface.
public interface ICharQ {
// Put a characer into the queue.
void put(char ch);
// Get a character from the queue.
char get();
}
// An exception for queue-full errors.
class QueueFullException : ApplicationException {
public QueueFullException() : base() { }
public QueueFullException(string str) : base(str) { }
public override string ToString() {
return "\n" + Message;
}
}
// An exception for queue-empty errors.
class QueueEmptyException : ApplicationException {
public QueueEmptyException() : base() { }
public QueueEmptyException(string str) : base(str) { }
public override string ToString() {
return "\n" + Message;
}
}
// A fixed-size queue class for characters that uses exceptions.
class FixedQueue : ICharQ {
char[] q; // this array holds the queue
int putloc, getloc; // the put and get indices
// Construct an empty queue given its size.
public FixedQueue(int size) {
q = new char[size+1]; // allocate memory for queue
putloc = getloc = 0;
}
// Put a character into the queue.
public void put(char ch) {
if(putloc==q.Length-1)
throw new QueueFullException("Max length is " +
(q.Length-1));
putloc++;
q[putloc] = ch;
}
// Get a character from the queue.
public char get() {
if(getloc == putloc)
throw new QueueEmptyException();
getloc++;
return q[getloc];
}
}
// Demonstrate the queue exceptions.
public class QExcDemo {
public static void Main() {
FixedQueue q = new FixedQueue(10);
char ch;
int i;
try {
// overrun the queue
for(i=0; i < 11; i++) {
Console.Write("Attempting to store : " +
(char) ('A' + i));
q.put((char) ('A' + i));
Console.WriteLine(" -- OK");
}
Console.WriteLine();
}
catch (QueueFullException exc) {
Console.WriteLine(exc);
}
Console.WriteLine();
try {
// over-empty the queue
for(i=0; i < 11; i++) {
Console.Write("Getting next char: ");
ch = q.get();
Console.WriteLine(ch);
}
}
catch (QueueEmptyException exc) {
Console.WriteLine(exc);
}
}
}
Related examples in the same category