Demonstrate the Queue class
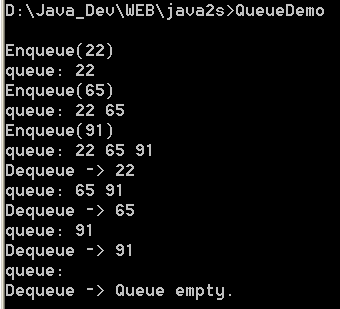
/*
C#: The Complete Reference
by Herbert Schildt
Publisher: Osborne/McGraw-Hill (March 8, 2002)
ISBN: 0072134852
*/
// Demonstrate the Queue class.
using System;
using System.Collections;
public class QueueDemo {
static void showEnq(Queue q, int a) {
q.Enqueue(a);
Console.WriteLine("Enqueue(" + a + ")");
Console.Write("queue: ");
foreach(int i in q)
Console.Write(i + " ");
Console.WriteLine();
}
static void showDeq(Queue q) {
Console.Write("Dequeue -> ");
int a = (int) q.Dequeue();
Console.WriteLine(a);
Console.Write("queue: ");
foreach(int i in q)
Console.Write(i + " ");
Console.WriteLine();
}
public static void Main() {
Queue q = new Queue();
foreach(int i in q)
Console.Write(i + " ");
Console.WriteLine();
showEnq(q, 22);
showEnq(q, 65);
showEnq(q, 91);
showDeq(q);
showDeq(q);
showDeq(q);
try {
showDeq(q);
} catch (InvalidOperationException) {
Console.WriteLine("Queue empty.");
}
}
}
Related examples in the same category