A queue class for characters
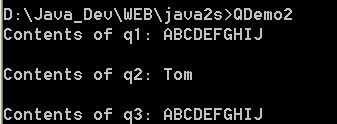
/*
C# A Beginner's Guide
By Schildt
Publisher: Osborne McGraw-Hill
ISBN: 0072133295
*/
// A queue class for characters.
using System;
class Queue {
// these are now private
char[] q; // this array holds the queue
int putloc, getloc; // the put and get indices
// Construct an empty Queue given its size.
public Queue(int size) {
q = new char[size+1]; // allocate memory for queue
putloc = getloc = 0;
}
// Construct a Queue from a Queue.
public Queue(Queue ob) {
putloc = ob.putloc;
getloc = ob.getloc;
q = new char[ob.q.Length];
// copy elements
for(int i=getloc+1; i <= putloc; i++)
q[i] = ob.q[i];
}
// Construct a Queue with initial values.
public Queue(char[] a) {
putloc = 0;
getloc = 0;
q = new char[a.Length+1];
for(int i = 0; i < a.Length; i++) put(a[i]);
}
// Put a character into the queue.
public void put(char ch) {
if(putloc==q.Length-1) {
Console.WriteLine(" -- Queue is full.");
return;
}
putloc++;
q[putloc] = ch;
}
// Get a character from the queue.
public char get() {
if(getloc == putloc) {
Console.WriteLine(" -- Queue is empty.");
return (char) 0;
}
getloc++;
return q[getloc];
}
}
// Demonstrate the Queue class.
public class QDemo2 {
public static void Main() {
// construct 10-element empty queue
Queue q1 = new Queue(10);
char[] name = {'T', 'o', 'm'};
// construct queue from array
Queue q2 = new Queue(name);
char ch;
int i;
// put some characters into q1
for(i=0; i < 10; i++)
q1.put((char) ('A' + i));
// construct queue from another queue
Queue q3 = new Queue(q1);
// Show the queues.
Console.Write("Contents of q1: ");
for(i=0; i < 10; i++) {
ch = q1.get();
Console.Write(ch);
}
Console.WriteLine("\n");
Console.Write("Contents of q2: ");
for(i=0; i < 3; i++) {
ch = q2.get();
Console.Write(ch);
}
Console.WriteLine("\n");
Console.Write("Contents of q3: ");
for(i=0; i < 10; i++) {
ch = q3.get();
Console.Write(ch);
}
}
}
Related examples in the same category