illustrates the use of BitArray methods
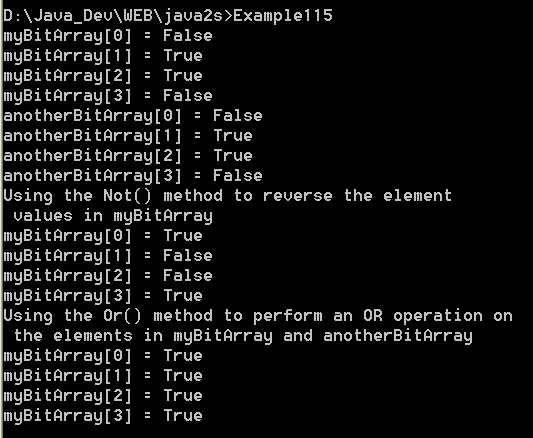
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example11_5.cs illustrates the use of BitArray methods
*/
using System;
using System.Collections;
public class Example11_5
{
// the DisplayBitArray() method displays the elements in the
// supplied BitArray
public static void DisplayBitArray(
string arrayListName, BitArray myBitArray
)
{
for (int counter = 0; counter < myBitArray.Count; counter++)
{
Console.WriteLine(arrayListName + "[" + counter + "] = " +
myBitArray[counter]);
}
}
public static void Main()
{
// create a BitArray object
BitArray myBitArray = new BitArray(4);
myBitArray[0] = false;
myBitArray[1] = true;
myBitArray[2] = true;
myBitArray[3] = false;
DisplayBitArray("myBitArray", myBitArray);
// create another BitArray object, passing myBitArray to
// the constructor
BitArray anotherBitArray = new BitArray(myBitArray);
DisplayBitArray("anotherBitArray", myBitArray);
// use the Not() method to reverse the elements in myBitArray
Console.WriteLine("Using the Not() method to reverse the element\n" +
" values in myBitArray");
myBitArray.Not();
DisplayBitArray("myBitArray", myBitArray);
// use the Or() method to perform an OR operation on the elements
// in myBitArray and anotherBitArray
Console.WriteLine("Using the Or() method to perform an OR operation on\n" +
" the elements in myBitArray and anotherBitArray");
myBitArray.Or(anotherBitArray);
DisplayBitArray("myBitArray", myBitArray);
}
}
Related examples in the same category