Get Selected Node Full Path
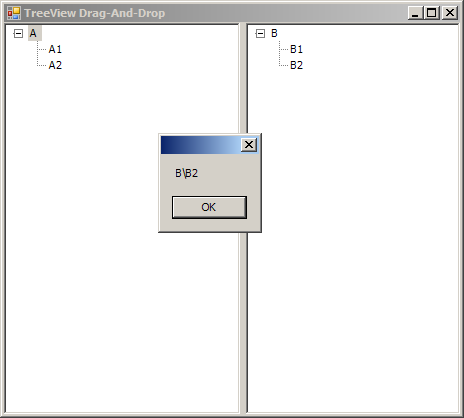
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
public class Form1 : Form
{
private System.Windows.Forms.SplitContainer splitContainer1;
private System.Windows.Forms.TreeView treeOne;
private System.Windows.Forms.TreeView treeTwo;
public Form1() {
InitializeComponent();
TreeNode node = treeOne.Nodes.Add("A");
node.Nodes.Add("A1");
node.Nodes.Add("A2");
node.Expand();
node = treeTwo.Nodes.Add("B");
node.Nodes.Add("B1");
node.Nodes.Add("B2");
node.Expand();
}
private void tree_DoubleClick(object sender, EventArgs e)
{
MessageBox.Show(((TreeView)sender).SelectedNode.FullPath);
}
private void InitializeComponent()
{
this.splitContainer1 = new System.Windows.Forms.SplitContainer();
this.treeOne = new System.Windows.Forms.TreeView();
this.treeTwo = new System.Windows.Forms.TreeView();
this.splitContainer1.Panel1.SuspendLayout();
this.splitContainer1.Panel2.SuspendLayout();
this.splitContainer1.SuspendLayout();
this.SuspendLayout();
//
// splitContainer1
//
this.splitContainer1.Dock = System.Windows.Forms.DockStyle.Fill;
this.splitContainer1.Location = new System.Drawing.Point(0, 0);
this.splitContainer1.Name = "splitContainer1";
//
// splitContainer1.Panel1
//
this.splitContainer1.Panel1.Controls.Add(this.treeOne);
//
// splitContainer1.Panel2
//
this.splitContainer1.Panel2.Controls.Add(this.treeTwo);
this.splitContainer1.Size = new System.Drawing.Size(456, 391);
this.splitContainer1.SplitterDistance = 238;
this.splitContainer1.TabIndex = 0;
this.splitContainer1.Text = "splitContainer1";
//
// treeOne
//
this.treeOne.Dock = System.Windows.Forms.DockStyle.Left;
this.treeOne.HideSelection = false;
this.treeOne.Location = new System.Drawing.Point(0, 0);
this.treeOne.Name = "treeOne";
this.treeOne.Size = new System.Drawing.Size(236, 391);
this.treeOne.TabIndex = 5;
this.treeOne.DoubleClick += new System.EventHandler(this.tree_DoubleClick);
//
// treeTwo
//
this.treeTwo.Dock = System.Windows.Forms.DockStyle.Fill;
this.treeTwo.Location = new System.Drawing.Point(0, 0);
this.treeTwo.Name = "treeTwo";
this.treeTwo.Size = new System.Drawing.Size(214, 391);
this.treeTwo.TabIndex = 7;
this.treeTwo.DoubleClick += new System.EventHandler(this.tree_DoubleClick);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(456, 391);
this.Controls.Add(this.splitContainer1);
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.Name = "Form1";
this.Text = "TreeView Drag-And-Drop";
this.splitContainer1.Panel1.ResumeLayout(false);
this.splitContainer1.Panel2.ResumeLayout(false);
this.splitContainer1.ResumeLayout(false);
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.Run(new Form1());
}
}
Related examples in the same category