A Generic Class with Two Type Parameters
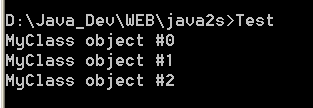
using System;
class MyGenericClass<T, V> {
T ob1;
V ob2;
public MyGenericClass(T o1, V o2) {
ob1 = o1;
ob2 = o2;
}
public void showTypes() {
Console.WriteLine("Type of T is " + typeof(T));
Console.WriteLine("Type of V is " + typeof(V));
}
public T getob1() {
return ob1;
}
public V getob2() {
return ob2;
}
}
public class Test {
public static void Main() {
MyGenericClass<int, string> obj = new MyGenericClass<int, string>(1, "string");
obj.showTypes();
int v = obj.getob1();
Console.WriteLine("value: " + v);
string str = obj.getob2();
Console.WriteLine("value: " + str);
}
}
Related examples in the same category