Generic class with interface
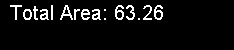
using System;
using System.Collections.Generic;
public interface IShape
{
double Area {
get;
}
}
public class Circle : IShape
{
public Circle( double radius ) {
this.radius = radius;
}
public double Area {
get {
return 3.14 * radius * radius;
}
}
private double radius;
}
public class Rect : IShape
{
public Rect( double width, double height ) {
this.width = width;
this.height = height;
}
public double Area {
get {
return width*height;
}
}
private double width;
private double height;
}
public class Shapes<T>
where T: IShape
{
public double TotalArea {
get {
double acc = 0;
foreach( T shape in shapes ) {
acc += shape.Area;
}
return acc;
}
}
public void Add( T shape ) {
shapes.Add( shape );
}
private List<T> shapes = new List<T>();
}
public class Test
{
static void Main() {
Shapes<IShape> shapes = new Shapes<IShape>();
shapes.Add( new Circle(3) );
shapes.Add( new Rect(7, 5) );
Console.WriteLine( "Total Area: {0}", shapes.TotalArea );
}
}
Related examples in the same category