Comparing Instances of a Type Parameter
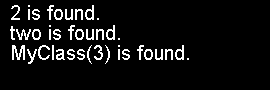
using System;
class MyClass : IComparable {
public int val;
public MyClass(int x) {
val = x;
}
public int CompareTo(object obj) {
return val - ((MyClass) obj).val;
}
}
class CompareDemo {
public static bool contains<T>(T what, T[] obs) where T : IComparable {
foreach(T v in obs)
if(v.CompareTo(what) == 0)
return true;
return false;
}
public static void Main() {
int[] nums = { 1, 2, 3, 4, 5 };
if(contains(2, nums))
Console.WriteLine("2 is found.");
string[] strs = { "one", "two", "three"};
if(contains("two", strs))
Console.WriteLine("two is found.");
MyClass[] mcs = { new MyClass(1), new MyClass(2),
new MyClass(3), new MyClass(4) };
if(contains(new MyClass(3), mcs))
Console.WriteLine("MyClass(3) is found.");
}
}
Related examples in the same category