Binds a TabControl to a collection of Employee objects
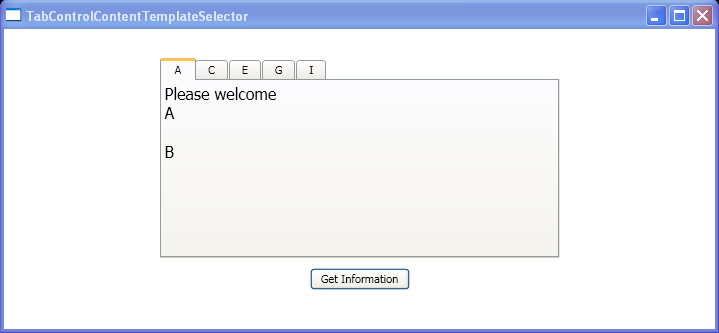
<Window x:Class="TabControlContentTemplateSelector.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:src="clr-namespace:TabControlContentTemplateSelector"
Title="TabControlContentTemplateSelector">
<Window.Resources>
<src:People x:Key="MyFriends"/>
<DataTemplate x:Key="DetailTemplate">
<StackPanel>
<TextBlock Text="First Name:"/>
<TextBlock Text="{Binding Path=FirstName}"/>
<TextBlock Text="Last Name:"/>
<TextBlock Text="{Binding Path=LastName}"/>
</StackPanel>
</DataTemplate>
<DataTemplate x:Key="SeattleTemplate">
<DataTemplate.Resources>
<Style TargetType="TextBlock">
<Setter Property="FontSize" Value="16"/>
</Style>
</DataTemplate.Resources>
<StackPanel>
<TextBlock Text="Please welcome"/>
<TextBlock Text="{Binding Path=FirstName}"/>
<TextBlock Text=" "/>
<TextBlock Text="{Binding Path=LastName}"/>
</StackPanel>
</DataTemplate>
<src:EmployeeTemplateSelector x:Key="EmployeeSelector"/>
</Window.Resources>
<StackPanel VerticalAlignment="Center">
<TabControl Name="tabCtrl1" Width="400" Height="200"
ItemsSource="{Binding Source={StaticResource MyFriends}}"
ContentTemplateSelector="{StaticResource EmployeeSelector}"/>
<Button Width="100" Margin="10" Name="infoBtn"
Click="infoBtn_Click">
_Get Information
</Button>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.ComponentModel;
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Media;
using System.Windows.Shapes;
using System.Collections.ObjectModel;
namespace TabControlContentTemplateSelector
{
public class Employee : INotifyPropertyChanged
{
private string firstname;
private string lastname;
public event PropertyChangedEventHandler PropertyChanged;
public Employee()
{
}
public Employee(string first, string last)
{
this.firstname = first;
this.lastname = last;
}
public override string ToString()
{
return firstname.ToString();
}
public string FirstName
{
get { return firstname; }
set
{
firstname = value;
OnPropertyChanged("FirstName");
}
}
public string LastName
{
get { return lastname; }
set
{
lastname = value;
OnPropertyChanged("LastName");
}
}
protected void OnPropertyChanged(string info)
{
PropertyChangedEventHandler handler = PropertyChanged;
if (handler != null)
{
handler(this, new PropertyChangedEventArgs(info));
}
}
}
public class People : ObservableCollection<Employee>
{
public People()
: base()
{
Add(new Employee("A", "B"));
Add(new Employee("C", "D"));
Add(new Employee("E", "F"));
Add(new Employee("G", "H"));
Add(new Employee("I", "J"));
}
}
public partial class Window1 : System.Windows.Window
{
public Window1()
{
InitializeComponent();
}
void infoBtn_Click(object sender, RoutedEventArgs e)
{
if (tabCtrl1.SelectedContent is Employee)
{
Employee selectedEmployee = tabCtrl1.SelectedContent as Employee;
StringBuilder personInfo = new StringBuilder();
personInfo.Append(selectedEmployee.FirstName);
personInfo.Append(" ");
personInfo.Append(selectedEmployee.LastName);
MessageBox.Show(personInfo.ToString());
}
}
}
public class EmployeeTemplateSelector : DataTemplateSelector
{
public override DataTemplate SelectTemplate(object item, DependencyObject container)
{
if (item is Employee)
{
Employee person = item as Employee;
Window win = Application.Current.MainWindow;
return win.FindResource("SeattleTemplate") as DataTemplate;
//return win.FindResource("DetailTemplate") as DataTemplate;
}
return null;
}
}
}
Related examples in the same category