A display of text, formatted by us instead of by AWT/Swing
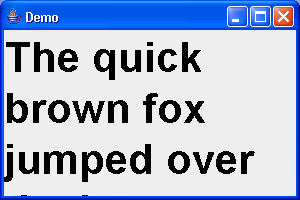
/*
* Copyright (c) Ian F. Darwin, http://www.darwinsys.com/, 1996-2002.
* All rights reserved. Software written by Ian F. Darwin and others.
* $Id: LICENSE,v 1.8 2004/02/09 03:33:38 ian Exp $
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR AND CONTRIBUTORS ``AS IS''
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED
* TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR OR CONTRIBUTORS
* BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* Java, the Duke mascot, and all variants of Sun's Java "steaming coffee
* cup" logo are trademarks of Sun Microsystems. Sun's, and James Gosling's,
* pioneering role in inventing and promulgating (and standardizing) the Java
* language and environment is gratefully acknowledged.
*
* The pioneering role of Dennis Ritchie and Bjarne Stroustrup, of AT&T, for
* inventing predecessor languages C and C++ is also gratefully acknowledged.
*/
import java.awt.Component;
import java.awt.Container;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Point;
import java.awt.font.FontRenderContext;
import java.awt.font.LineBreakMeasurer;
import java.awt.font.TextAttribute;
import java.awt.font.TextLayout;
import java.text.AttributedString;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import javax.swing.JFrame;
/**
* A display of text, formatted by us instead of by AWT/Swing.
* <P>
* This program is distributed under the terms of the accompanying COPYRIGHT.txt
* file (which is NOT the GNU General Public License). Please read it. Your use
* of the software constitutes acceptance of the terms in the COPYRIGHT.txt
* file.
*
* @author Ian F. Darwin
* @version $Id: TextFormat.java,v 1.3 2004/03/26 03:34:30 ian Exp $
*/
public class TextFormat extends Component {
/** The text of this line */
protected String text;
/** The Font */
protected Font font;
/** The TextLayouts corresponding to "text" */
List layouts;
public Font getFont() {
return font;
}
public void setFont(Font f) {
font = f;
}
public String getText() {
return text;
}
public void setText(String t) {
text = t;
}
public void paint(Graphics g) {
if (text == null || text.length() == 0)
return;
if (layouts == null)
getLayouts(g);
Point pen = new Point(0, 0);
Graphics2D g2d = (Graphics2D) g;
g2d.setColor(java.awt.Color.black); // or a property
g2d.setFont(font);
Iterator it = layouts.iterator();
while (it.hasNext()) {
TextLayout layout = (TextLayout) it.next();
pen.y += (layout.getAscent());
g2d.setFont(font);
layout.draw(g2d, pen.x, pen.y);
pen.y += layout.getDescent();
//pen.y += leading;
}
}
/**
* Lazy evaluation of the List of TextLayout objects corresponding to this
* MText. Some things are approximations!
*/
private void getLayouts(Graphics g) {
layouts = new ArrayList();
Point pen = new Point(10, 20);
Graphics2D g2d = (Graphics2D) g;
FontRenderContext frc = g2d.getFontRenderContext();
AttributedString attrStr = new AttributedString(text);
attrStr.addAttribute(TextAttribute.FONT, font, 0, text.length());
LineBreakMeasurer measurer = new LineBreakMeasurer(attrStr
.getIterator(), frc);
float wrappingWidth;
wrappingWidth = getSize().width - 15;
while (measurer.getPosition() < text.length()) {
TextLayout layout = measurer.nextLayout(wrappingWidth);
layouts.add(layout);
}
}
public static void main(String[] args) {
JFrame jf = new JFrame("Demo");
Container cp = jf.getContentPane();
TextFormat tl = new TextFormat();
tl.setFont(new Font("SansSerif", Font.BOLD, 42));
tl.setText("The quick brown fox jumped over the lazy cow");
cp.add(tl);
jf.setSize(300, 200);
jf.setVisible(true);
}
}
Related examples in the same category