Caret action
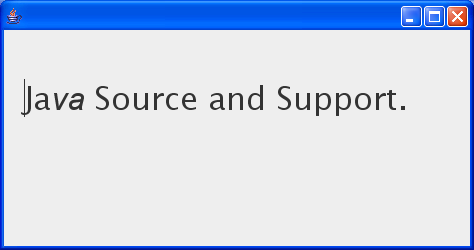
import java.awt.BasicStroke;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.RenderingHints;
import java.awt.Shape;
import java.awt.Stroke;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.awt.font.FontRenderContext;
import java.awt.font.TextAttribute;
import java.awt.font.TextHitInfo;
import java.awt.font.TextLayout;
import java.awt.geom.AffineTransform;
import java.text.AttributedCharacterIterator;
import java.text.AttributedString;
import javax.swing.JFrame;
import javax.swing.JLabel;
public class TextLayoutWithCarets extends JLabel {
private TextHitInfo mHit;
private TextLayout mLayout;
private boolean mInitialized = false;
public TextLayoutWithCarets() {
addKeyListener(new KeyListener() {
public void keyPressed(KeyEvent ke) {
if (ke.getKeyCode() == KeyEvent.VK_RIGHT) {
mHit = mLayout.getNextRightHit(mHit.getInsertionIndex());
if (mHit == null)
mHit = mLayout.getNextLeftHit(1);
repaint();
} else if (ke.getKeyCode() == KeyEvent.VK_LEFT) {
mHit = mLayout.getNextLeftHit(mHit.getInsertionIndex());
if (mHit == null)
mHit = mLayout.getNextRightHit(mLayout
.getCharacterCount() - 1);
repaint();
}
}
public void keyTyped(KeyEvent arg0) {
// TODO Auto-generated method stub
}
public void keyReleased(KeyEvent arg0) {
// TODO Auto-generated method stub
}
});
}
private void initialize(Graphics2D g2) {
String s = "Java Source and Support.";
// Create a plain and italic font.
int fontSize = 32;
Font font = new Font("Lucida Sans Regular", Font.PLAIN, fontSize);
Font italicFont = new Font("Lucida Sans Oblique", Font.ITALIC, fontSize);
// Create an Attributed String
AttributedString as = new AttributedString(s);
as.addAttribute(TextAttribute.FONT, font);
as.addAttribute(TextAttribute.FONT, italicFont, 2, 5);
// Get the iterator.
AttributedCharacterIterator iterator = as.getIterator();
// Create a TextLayout.
FontRenderContext frc = g2.getFontRenderContext();
mLayout = new TextLayout(iterator, frc);
mHit = mLayout.getNextLeftHit(1);
// Respond to left and right arrow keys.
mInitialized = true;
}
public void paint(Graphics g) {
Graphics2D g2 = (Graphics2D) g;
g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING,
RenderingHints.VALUE_ANTIALIAS_ON);
g2.setRenderingHint(RenderingHints.KEY_FRACTIONALMETRICS,
RenderingHints.VALUE_FRACTIONALMETRICS_ON);
if (mInitialized == false)
initialize(g2);
float x = 20, y = 80;
mLayout.draw(g2, x, y);
// Create a plain stroke and a dashed stroke.
Stroke[] caretStrokes = new Stroke[2];
caretStrokes[0] = new BasicStroke();
caretStrokes[1] = new BasicStroke(1, BasicStroke.CAP_BUTT,
BasicStroke.JOIN_ROUND, 0, new float[] { 4, 4 }, 0);
// Now draw the carets
Shape[] carets = mLayout.getCaretShapes(mHit.getInsertionIndex());
for (int i = 0; i < carets.length; i++) {
if (carets[i] != null) {
AffineTransform at = AffineTransform.getTranslateInstance(x, y);
Shape shape = at.createTransformedShape(carets[i]);
g2.setStroke(caretStrokes[i]);
g2.draw(shape);
}
}
}
public static void main(String[] args) {
JFrame f = new JFrame();
f.getContentPane().add(new TextLayoutWithCarets());
f.setSize(350, 250);
f.setVisible(true);
}
}
Related examples in the same category