Unicode: test layout
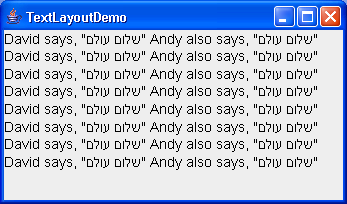
/*
Java Internationalization
By Andy Deitsch, David Czarnecki
ISBN: 0-596-00019-7
O'Reilly
*/
import java.awt.*;
import java.awt.event.*;
import java.awt.font.*;
import java.text.*;
import javax.swing.*;
public class TextLayoutDemo extends JFrame {
String davidMessage = "David says, \"\u05E9\u05DC\u05D5\u05DD \u05E2\u05D5\u05DC\u05DD\" ";
String andyMessage = "Andy also says, \"\u05E9\u05DC\u05D5\u05DD \u05E2\u05D5\u05DC\u05DD\" ";
String textMessage = davidMessage + andyMessage + davidMessage + andyMessage +
davidMessage + andyMessage + davidMessage + andyMessage +
davidMessage + andyMessage + davidMessage + andyMessage +
davidMessage + andyMessage + davidMessage + andyMessage;
public TextLayoutDemo() {
super("TextLayoutDemo");
}
public void paint(Graphics g) {
Graphics2D graphics2D = (Graphics2D)g;
GraphicsEnvironment.getLocalGraphicsEnvironment();
Font font = new Font("LucidaSans", Font.PLAIN, 14);
AttributedString messageAS = new AttributedString(textMessage);
messageAS.addAttribute(TextAttribute.FONT, font);
AttributedCharacterIterator messageIterator = messageAS.getIterator();
FontRenderContext messageFRC = graphics2D.getFontRenderContext();
LineBreakMeasurer messageLBM = new LineBreakMeasurer(messageIterator, messageFRC);
Insets insets = getInsets();
float wrappingWidth = getSize().width - insets.left - insets.right;
float x = insets.left;
float y = insets.top;
while (messageLBM.getPosition() < messageIterator.getEndIndex()) {
TextLayout textLayout = messageLBM.nextLayout(wrappingWidth);
y += textLayout.getAscent();
textLayout.draw(graphics2D, x, y);
y += textLayout.getDescent() + textLayout.getLeading();
x = insets.left;
}
}
public static void main(String[] args) {
JFrame frame = new TextLayoutDemo();
frame.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {System.exit(0);}
});
frame.pack();
frame.setVisible(true);
}
}
Related examples in the same category