Print Hello World in black, outlined in red, to default printer
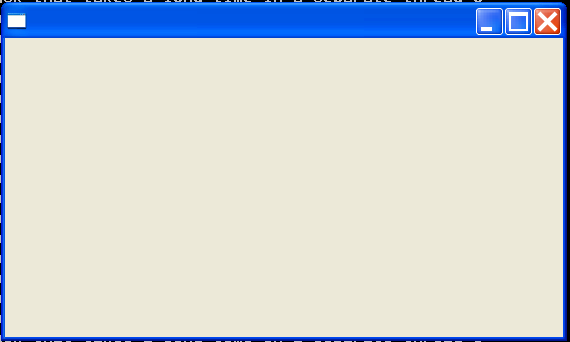
/*
* Printing example snippet: print "Hello World!" in black, outlined in red, to default printer
*
* For a list of all SWT example snippets see
* http://dev.eclipse.org/viewcvs/index.cgi/%7Echeckout%7E/platform-swt-home/dev.html#snippets
*/
import org.eclipse.swt.SWT;
import org.eclipse.swt.graphics.Color;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.graphics.GC;
import org.eclipse.swt.graphics.Point;
import org.eclipse.swt.graphics.Rectangle;
import org.eclipse.swt.printing.Printer;
import org.eclipse.swt.printing.PrinterData;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class Snippet132 {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.open();
PrinterData data = Printer.getDefaultPrinterData();
if (data == null) {
System.out.println("Warning: No default printer.");
return;
}
Printer printer = new Printer(data);
if (printer.startJob("SWT Printing Snippet")) {
Color black = printer.getSystemColor(SWT.COLOR_BLACK);
Color white = printer.getSystemColor(SWT.COLOR_WHITE);
Color red = printer.getSystemColor(SWT.COLOR_RED);
Rectangle trim = printer.computeTrim(0, 0, 0, 0);
Point dpi = printer.getDPI();
int leftMargin = dpi.x + trim.x; // one inch from left side of
// paper
int topMargin = dpi.y / 2 + trim.y; // one-half inch from top edge
// of paper
GC gc = new GC(printer);
Font font = gc.getFont(); // example just uses printer's default
// font
if (printer.startPage()) {
gc.setBackground(white);
gc.setForeground(black);
String testString = "Hello World!";
Point extent = gc.stringExtent(testString);
gc.drawString(testString, leftMargin, topMargin
+ font.getFontData()[0].getHeight());
gc.setForeground(red);
gc.drawRectangle(leftMargin, topMargin, extent.x, extent.y);
printer.endPage();
}
gc.dispose();
printer.endJob();
}
printer.dispose();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Related examples in the same category