JGoodies Binding: Bean Adapter Example
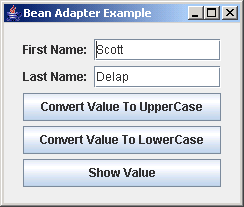
/*
Code revised from Desktop Java Live:
http://www.sourcebeat.com/downloads/
*/
import java.awt.event.ActionEvent;
import javax.swing.AbstractAction;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
import com.jgoodies.binding.adapter.BasicComponentFactory;
import com.jgoodies.binding.beans.BeanAdapter;
import com.jgoodies.binding.beans.Model;
import com.jgoodies.binding.value.ValueModel;
import com.jgoodies.forms.builder.DefaultFormBuilder;
import com.jgoodies.forms.layout.FormLayout;
public class BeanAdapterExample extends JPanel {
private PersonBean personBean;
public BeanAdapterExample() {
DefaultFormBuilder defaultFormBuilder = new DefaultFormBuilder(new FormLayout("p, 2dlu, p:g"));
defaultFormBuilder.setDefaultDialogBorder();
this.personBean = new PersonBean("Scott", "Delap");
BeanAdapter beanAdapter = new BeanAdapter(this.personBean, true);
ValueModel firstNameAdapter = beanAdapter.getValueModel("firstName");
ValueModel lastNameAdapter = beanAdapter.getValueModel("lastName");
JTextField firstNameTextField = BasicComponentFactory.createTextField(firstNameAdapter);
JTextField lastNameTextField = BasicComponentFactory.createTextField(lastNameAdapter);
defaultFormBuilder.append("First Name: ", firstNameTextField);
defaultFormBuilder.append("Last Name: ", lastNameTextField);
defaultFormBuilder.append(new JButton(new ConvertValueToUpperCaseAction()), 3);
defaultFormBuilder.append(new JButton(new ConvertValueToLowerCaseAction()), 3);
defaultFormBuilder.append(new JButton(new ShowValueHolderValueAction()), 3);
add(defaultFormBuilder.getPanel());
}
private class ConvertValueToUpperCaseAction extends AbstractAction {
public ConvertValueToUpperCaseAction() {
super("Convert Value To UpperCase");
}
public void actionPerformed(ActionEvent event) {
personBean.setFirstName(personBean.getFirstName().toUpperCase());
personBean.setLastName(personBean.getLastName().toUpperCase());
}
}
private class ConvertValueToLowerCaseAction extends AbstractAction {
public ConvertValueToLowerCaseAction() {
super("Convert Value To LowerCase");
}
public void actionPerformed(ActionEvent event) {
personBean.setFirstName(personBean.getFirstName().toLowerCase());
personBean.setLastName(personBean.getLastName().toLowerCase());
}
}
private class ShowValueHolderValueAction extends AbstractAction {
public ShowValueHolderValueAction() {
super("Show Value");
}
public void actionPerformed(ActionEvent event) {
StringBuffer message = new StringBuffer();
message.append("<html>");
message.append("<b>First Name:</b> ");
message.append(personBean.getFirstName());
message.append("<br><b>Last Name:</b> ");
message.append(personBean.getLastName());
message.append("</html>");
JOptionPane.showMessageDialog(null, message.toString());
}
}
public class PersonBean extends Model {
private String firstName;
private String lastName;
public static final String FIRST_NAME_PROPERTY = "firstName";
public static final String LAST_NAME_PROPERTY = "lastName";
public PersonBean(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
String oldValue = this.firstName;
this.firstName = firstName;
firePropertyChange(FIRST_NAME_PROPERTY, oldValue, this.firstName);
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
String oldValue = this.lastName;
this.lastName = lastName;
firePropertyChange(LAST_NAME_PROPERTY, oldValue, this.lastName);
}
}
public static void main(String[] a){
JFrame f = new JFrame("Bean Adapter Example");
f.setDefaultCloseOperation(2);
f.add(new BeanAdapterExample());
f.pack();
f.setVisible(true);
}
}
jgoodiesDataBinding.zip( 254 k)Related examples in the same category