JGoodies Binding: Feed Bean Example 2
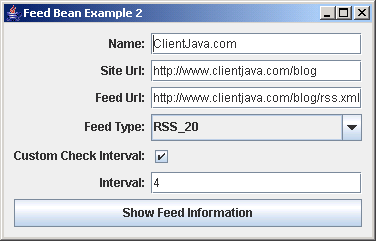
/*
Code revised from Desktop Java Live:
http://www.sourcebeat.com/downloads/
*/
import java.awt.event.ActionEvent;
import java.util.Arrays;
import java.util.List;
import javax.swing.AbstractAction;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JCheckBox;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
import com.jgoodies.forms.builder.DefaultFormBuilder;
import com.jgoodies.forms.layout.FormLayout;
public class FeedBeanExample2 extends JPanel {
JTextField nameField;
JTextField siteField;
JTextField feedField;
JComboBox feedTypesComboBox;
JCheckBox customCheckIntervalCheckbox;
JTextField intervalField;
Feed feed;
public FeedBeanExample2() {
DefaultFormBuilder formBuilder = new DefaultFormBuilder(new FormLayout(""));
formBuilder.setBorder(BorderFactory.createEmptyBorder(5, 5, 5, 5));
formBuilder.appendColumn("right:pref");
formBuilder.appendColumn("3dlu");
formBuilder.appendColumn("fill:p:g");
this.nameField = new JTextField();
formBuilder.append("Name:", this.nameField);
this.siteField = new JTextField();
formBuilder.append("Site Url:", this.siteField);
this.feedField = new JTextField();
formBuilder.append("Feed Url:", this.feedField);
this.feedTypesComboBox = new JComboBox(Arrays.asList(new FeedType[]{
new FeedType("RSS_09"), new FeedType("RSS_093"), new FeedType("RSS_20"),
new FeedType("ATOM_03")}).toArray());
formBuilder.append("Feed Type:", this.feedTypesComboBox);
this.customCheckIntervalCheckbox = new JCheckBox();
formBuilder.append("Custom Check Interval:", this.customCheckIntervalCheckbox);
this.intervalField = new JTextField();
formBuilder.append("Interval:", this.intervalField);
formBuilder.append(new JButton(new ShowFeedInformationAction()), 3);
createFeed();
initializeFormElements();
add(formBuilder.getPanel());
}
private void createFeed() {
this.feed = new Feed();
this.feed.setName("ClientJava.com");
this.feed.setSiteUrl("http://www.clientjava.com/blog");
this.feed.setFeedUrl("http://www.clientjava.com/blog/rss.xml");
this.feed.setFeedType(new FeedType("RSS_20"));
this.feed.setCustomCheckIntervalEnabled(true);
this.feed.setCheckInterval(new Integer(4));
}
private void initializeFormElements() {
this.nameField.setText(this.feed.getName());
this.siteField.setText(this.feed.getSiteUrl());
this.feedField.setText(this.feed.getFeedUrl());
this.feedTypesComboBox.setSelectedItem(this.feed.getFeedType());
this.customCheckIntervalCheckbox.setSelected(this.feed.isCustomCheckIntervalEnabled());
if (this.feed.getCheckInterval() != null) {
this.intervalField.setText(this.feed.getCheckInterval().toString());
}
}
private void synchFormAndFeed() {
this.feed.setName(this.nameField.getText());
this.feed.setSiteUrl(this.siteField.getText());
this.feed.setFeedUrl(this.feedField.getText());
this.feed.setFeedType((FeedType) this.feedTypesComboBox.getSelectedItem());
this.feed.setCustomCheckIntervalEnabled(this.customCheckIntervalCheckbox.isSelected());
if (this.feed.isCustomCheckIntervalEnabled()) {
this.feed.setCheckInterval(new Integer(this.intervalField.getText()));
} else {
this.feed.setCheckInterval(null);
}
}
private Feed getFeed() {
return this.feed;
}
private class ShowFeedInformationAction extends AbstractAction {
public ShowFeedInformationAction() {
super("Show Feed Information");
}
public void actionPerformed(ActionEvent event) {
synchFormAndFeed();
StringBuffer message = new StringBuffer();
message.append("<html>");
message.append("<b>Name:</b> ");
message.append(getFeed().getName());
message.append("<br>");
message.append("<b>Site URL:</b> ");
message.append(getFeed().getSiteUrl());
message.append("<br>");
message.append("<b>Feed URL:</b> ");
message.append(getFeed().getFeedUrl());
message.append("<br>");
message.append("<b>Feed Type:</b> ");
message.append(getFeed().getFeedType());
message.append("<br>");
message.append("<b>Custom Check:</b> ");
message.append(getFeed().isCustomCheckIntervalEnabled());
message.append("<br>");
message.append("<b>Check Interval:</b> ");
message.append(getFeed().getCheckInterval());
message.append("</html>");
JOptionPane.showMessageDialog(null, message.toString());
}
}
public static void main(String[] a){
JFrame f = new JFrame("Feed Bean Example 2");
f.setDefaultCloseOperation(2);
f.add(new FeedBeanExample2());
f.pack();
f.setVisible(true);
}
class Feed {
private long id = 0;
private String name;
private String siteUrl;
private String feedUrl;
private FeedType feedType;
private boolean customCheckIntervalEnabled = false;
private Integer checkInterval;
public Feed() {
}
public Feed(String name, String siteUrl, String feedUrl, FeedType feedType) {
this.name = name;
this.siteUrl = siteUrl;
this.feedUrl = feedUrl;
this.feedType = feedType;
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String newName) {
this.name = newName;
}
public String getSiteUrl() {
return siteUrl;
}
public void setSiteUrl(String newSiteUrl) {
this.siteUrl = newSiteUrl;
}
public String getFeedUrl() {
return feedUrl;
}
public void setFeedUrl(String newFeedUrl) {
this.feedUrl = newFeedUrl;
}
public FeedType getFeedType() {
return feedType;
}
public void setFeedType(FeedType newFeedType) {
this.feedType = newFeedType;
}
public boolean isCustomCheckIntervalEnabled() {
return customCheckIntervalEnabled;
}
public void setCustomCheckIntervalEnabled(boolean newCustomCheckIntervalEnabled) {
this.customCheckIntervalEnabled = newCustomCheckIntervalEnabled;
}
public Integer getCheckInterval() {
return checkInterval;
}
public void setCheckInterval(Integer newCheckInterval) {
this.checkInterval = newCheckInterval;
}
public String toString() {
return this.name;
}
}
class FeedType {
private String type;
private FeedType(String type) {
this.type = type;
}
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof FeedType)) return false;
final FeedType feedType = (FeedType) o;
if (type != null ? !type.equals(feedType.type) : feedType.type != null) return false;
return true;
}
public int hashCode() {
return (type != null ? type.hashCode() : 0);
}
public String toString() {
return this.type;
}
}
}
jgoodiesDataBinding.zip( 254 k)Related examples in the same category