Trigger Race Animation
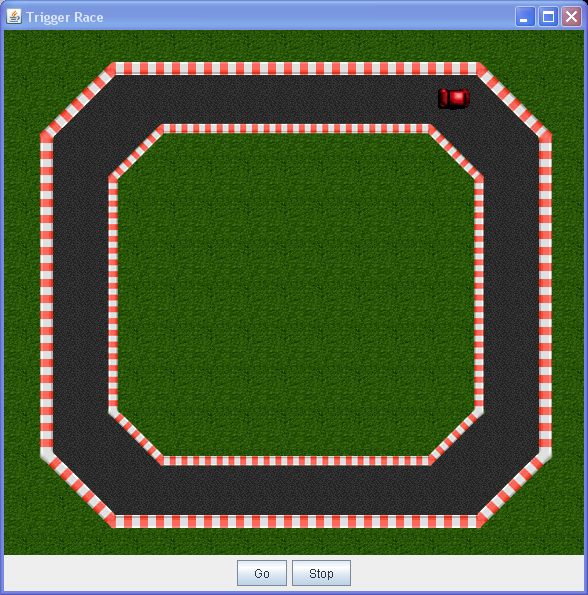
import javax.swing.SwingUtilities;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.SwingUtilities;
import org.jdesktop.animation.timing.Animator;
import org.jdesktop.animation.timing.TimingTargetAdapter;
import java.awt.event.ActionEvent;
import javax.swing.JButton;
import javax.swing.SwingUtilities;
import org.jdesktop.animation.timing.triggers.ActionTrigger;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import java.awt.BorderLayout;
import javax.swing.JFrame;
import javax.swing.UIManager;
import java.awt.AlphaComposite;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.awt.Point;
import javax.swing.JComponent;
import javax.imageio.ImageIO;
import javax.swing.JFrame;
/*
* TriggerRace.java
*
* Created on May 3, 2007, 1:43 PM
*
* Copyright (c) 2007, Sun Microsystems, Inc
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of the TimingFramework project nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/**
* Exactly like SetterRace, only this version uses Triggers to
* start/stop the animation automatically based on the user
* clicking the Go/Stop buttons (no need for an ActionListener here)
*
* @author Chet
*/
public class TriggerRace extends NonLinearRace {
/** Creates a new instance of TriggerRace */
public TriggerRace(String appName) {
super(appName);
// Clicks on the Go button will atuomatically start the animator
JButton goButton = controlPanel.getGoButton();
ActionTrigger trigger = ActionTrigger.addTrigger(goButton, animator);
}
/**
* Handle clicks on the Stop button. Clicks on Go are handled through
* the ActionTrigger above.
*/
public void actionPerformed(ActionEvent ae) {
if (ae.getActionCommand().equals("Stop")) {
animator.stop();
}
}
public static void main(String args[]) {
Runnable doCreateAndShowGUI = new Runnable() {
public void run() {
TriggerRace race = new TriggerRace("Trigger Race");
}
};
SwingUtilities.invokeLater(doCreateAndShowGUI);
}
}
/**
* Copyright (c) 2007, Sun Microsystems, Inc
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of the TimingFramework project nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/**
* This class does the work of rendering the current view of the
* racetrack. It holds the car position and rotation and displays
* the car accordingly. The track itself is merely a background image
* that is copied the same on every repaint.
* Note that carPosition and carRotation are both JavaBean properties, which
* is exploited in the SetterRace and MultiStepRace variations.
*
* @author Chet
*/
class TrackView extends JComponent {
BufferedImage car;
BufferedImage track;
Point carPosition;
double carRotation = 0;
int trackW, trackH;
int carW, carH, carWHalf, carHHalf;
/** Hard-coded positions of interest on the track */
static final Point START_POS = new Point(450, 70);
static final Point FIRST_TURN_START = new Point(130, 70);
static final Point FIRST_TURN_END = new Point(76, 127);
static final Point SECOND_TURN_START = new Point(76, 404);
static final Point SECOND_TURN_END = new Point(130, 461);
static final Point THIRD_TURN_START = new Point(450, 461);
static final Point THIRD_TURN_END = new Point(504, 404);
static final Point FOURTH_TURN_START = new Point(504, 127);
/** Creates a new instance of TrackView */
public TrackView() {
try {
car = ImageIO.read(TrackView.class.getResource("beetle_red.gif"));
track = ImageIO.read(TrackView.class.getResource("track.jpg"));
} catch (Exception e) {
System.out.println("Problem loading track/car images: " + e);
}
carPosition = new Point(START_POS.x, START_POS.y);
carW = car.getWidth();
carH = car.getHeight();
carWHalf = carW / 2;
carHHalf = carH / 2;
trackW = track.getWidth();
trackH = track.getHeight();
}
public Dimension getPreferredSize() {
return new Dimension(trackW, trackH);
}
/**
* Render the track and car
*/
public void paintComponent(Graphics g) {
// First draw the race track
g.drawImage(track, 0, 0, null);
// Now draw the car. The translate/rotate/translate settings account
// for any nonzero carRotation values
Graphics2D g2d = (Graphics2D)g.create();
g2d.translate(carPosition.x, carPosition.y);
g2d.rotate(Math.toRadians(carRotation));
g2d.translate(-(carPosition.x), -(carPosition.y));
// Now the graphics has been set up appropriately; draw the
// car in position
g2d.drawImage(car, carPosition.x - carWHalf, carPosition.y - carHHalf, null);
}
/**
* Set the new position and schedule a repaint
*/
public void setCarPosition(Point newPosition) {
repaint(0, carPosition.x - carWHalf, carPosition.y - carHHalf,
carW, carH);
carPosition.x = newPosition.x;
carPosition.y = newPosition.y;
repaint(0, carPosition.x - carWHalf, carPosition.y - carHHalf,
carW, carH);
}
/**
* Set the new rotation and schedule a repaint
*/
public void setCarRotation(double newDegrees) {
carRotation = newDegrees;
// repaint area accounts for larger rectangular are because rotate
// car will exceed normal rectangular bounds
repaint(0, carPosition.x - carW, carPosition.y - carH,
2 * carW, 2 * carH);
}
}
/**
* Copyright (c) 2007, Sun Microsystems, Inc
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of the TimingFramework project nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/**
* The GUI used by all of the different race demos.
* It contains a control panel (for the Go/Stop buttons) and a
* TrackView (where the race is rendered)
*
* @author Chet
*/
class RaceGUI {
private TrackView track;
private RaceControlPanel controlPanel;
/**
* Creates a new instance of RaceGUI
*/
public RaceGUI(String appName) {
UIManager.put("swing.boldMetal", Boolean.FALSE);
JFrame f = new JFrame(appName);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setLayout(new BorderLayout());
// Add Track view
track = new TrackView();
f.add(track, BorderLayout.CENTER);
// Add control panel
controlPanel = new RaceControlPanel();
f.add(controlPanel, BorderLayout.SOUTH);
f.pack();
f.setVisible(true);
}
public TrackView getTrack() {
return track;
}
public RaceControlPanel getControlPanel() {
return controlPanel;
}
}
/**
* Copyright (c) 2007, Sun Microsystems, Inc
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of the TimingFramework project nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/**
* Go/Stop buttons to control the animation
*
* @author Chet
*/
class RaceControlPanel extends JPanel {
/** Make these static so that outside classes can easily
* add themselves as listeners */
JButton goButton = new JButton("Go");
JButton stopButton = new JButton("Stop");
/**
* Creates a new instance of RaceControlPanel
*/
public RaceControlPanel() {
add(goButton);
add(stopButton);
}
public JButton getGoButton() {
return goButton;
}
public JButton getStopButton() {
return stopButton;
}
public void addListener(ActionListener listener) {
goButton.addActionListener(listener);
stopButton.addActionListener(listener);
}
}
/*
* NonLinearRace.java
*
* Created on May 3, 2007, 7:49 AM
*
* Copyright (c) 2007, Sun Microsystems, Inc
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of the TimingFramework project nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/**
* Simple subclass of SetterRace that merely sets acceleration/deceleration
* factors to give a non-linear motion effect to the car's movement.
*
* @author Chet
*/
class NonLinearRace extends BasicRace {
/** Creates a new instance of NonLinearRace */
public NonLinearRace(String appName) {
super(appName);
// Accelerate for first half of duration, decelerate for final 10%
animator.setAcceleration(.5f);
animator.setDeceleration(.1f);
}
public static void main(String args[]) {
Runnable doCreateAndShowGUI = new Runnable() {
public void run() {
NonLinearRace race = new NonLinearRace("Non-Linear Race");
}
};
SwingUtilities.invokeLater(doCreateAndShowGUI);
}
}
/*
* BasicRace.java
*
* Created on May 3, 2007, 7:37 AM
*
* Copyright (c) 2007, Sun Microsystems, Inc
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of the TimingFramework project nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/**
* The simplest version of the animation; set up a Animator to
* move the car from one position to another over a given time period.
*
*
* @author Chet
*/
class BasicRace extends TimingTargetAdapter implements ActionListener {
public static final int RACE_TIME = 2000;
Point start = TrackView.START_POS;
Point end = TrackView.FIRST_TURN_START;
Point current = new Point();
protected Animator animator;
TrackView track;
RaceControlPanel controlPanel;
/** Creates a new instance of BasicRace */
public BasicRace(String appName) {
RaceGUI basicGUI = new RaceGUI(appName);
controlPanel = basicGUI.getControlPanel();
controlPanel.addListener(this);
track = basicGUI.getTrack();
animator = new Animator(RACE_TIME, this);
}
//
// Events
//
/**
* This receives the Go/Stop events that start/stop the animation
*/
public void actionPerformed(ActionEvent ae) {
if (ae.getActionCommand().equals("Go")) {
animator.stop();
animator.start();
} else if (ae.getActionCommand().equals("Stop")) {
animator.stop();
}
}
/**
* TimingTarget implementation: calculate and set the current
* car position based on the animation fraction
*/
public void timingEvent(float fraction) {
// Simple linear interpolation to find current position
current.x = (int)(start.x + (end.x - start.x) * fraction);
current.y = (int)(start.y + (end.y - start.y) * fraction);
// set the new position; this will force a repaint in TrackView
// and will display the car in the new position
track.setCarPosition(current);
}
public static void main(String args[]) {
Runnable doCreateAndShowGUI = new Runnable() {
public void run() {
BasicRace race = new BasicRace("BasicRace");
}
};
SwingUtilities.invokeLater(doCreateAndShowGUI);
}
}
Filthy-Rich-Clients-TriggerRace.zip( 484 k)Related examples in the same category