Class with two constructors
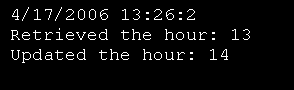
Imports System
Public Class MainClass
Shared Sub Main()
Dim currentTime As DateTime = DateTime.Now
Dim time1 As New Time(currentTime)
time1.DisplayCurrentTime( )
Dim theHour As Integer = time1.Hour
Console.WriteLine("Retrieved the hour: {0}", _
theHour)
theHour += 1
time1.Hour = theHour
Console.WriteLine("Updated the hour: {0}", _
time1.Hour)
End Sub
End Class
Public Class Time
Private mYear As Integer
Private mMonth As Integer
Private mDayOfMonth As Integer
Private mHour As Integer
Private mMinute As Integer
Private mSecond As Integer
Property Hour( ) As Integer
Get
Return mHour
End Get
Set(ByVal Value As Integer)
mHour = Value
End Set
End Property
' public accessor methods
Public Sub DisplayCurrentTime( )
Console.WriteLine( _
"{0}/{1}/{2} {3}:{4}:{5}", _
mMonth, mDayOfMonth, mYear, Hour, mMinute, mSecond)
End Sub 'DisplayCurrentTime
' constructors
Public Sub New(ByVal dt As DateTime)
mYear = dt.Year
mMonth = dt.Month
mDayOfMonth = dt.Day
mHour = dt.Hour
mMinute = dt.Minute
mSecond = dt.Second
End Sub 'New
Public Sub New( _
ByVal mYear As Integer, _
ByVal mMonth As Integer, _
ByVal mDayOfMonth As Integer, _
ByVal mHour As Integer, _
ByVal mMinute As Integer, _
ByVal mSecond As Integer)
Me.mYear = mYear
Me.mMonth = mMonth
Me.mDayOfMonth = mDayOfMonth
Me.Hour = mHour
Me.mMinute = mMinute
Me.mSecond = mSecond
End Sub 'New
End Class 'Time
Related examples in the same category