Overloading constructors
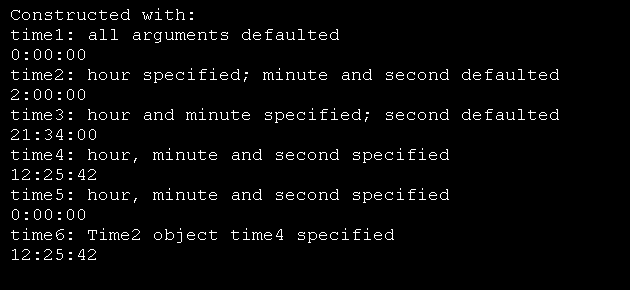
Imports System
Public Class MainClass
Shared Sub Main(ByVal args As String())
' use overloaded constructors
Dim time1 As New CTime2()
Dim time2 As New CTime2(2)
Dim time3 As New CTime2(21, 34)
Dim time4 As New CTime2(12, 25, 42)
Dim time5 As New CTime2(27, 74, 99)
Dim time6 As New CTime2(time4) ' use time4 as initial value
Const SPACING As Integer = 13 ' spacing between output text
Console.WriteLine( "Constructed with: " & vbCrLf & _
" time1: all arguments defaulted" & vbCrLf & _
Space(SPACING) & time1.ToUniversalString() )
' invoke time2 methods
Console.WriteLine( " time2: hour specified; minute and second defaulted" & _
vbCrLf & Space(SPACING) & _
time2.ToUniversalString() )
' invoke time3 methods
Console.WriteLine( " time3: hour and minute specified; second defaulted" & _
vbCrLf & Space(SPACING) & time3.ToUniversalString() )
' invoke time4 methods
Console.WriteLine( " time4: hour, minute and second specified" & _
vbCrLf & Space(SPACING) & time4.ToUniversalString() )
' invoke time5 methods
Console.WriteLine( " time5: hour, minute and second specified" & _
vbCrLf & Space(SPACING) & time5.ToUniversalString() )
' invoke time6 methods
Console.WriteLine( " time6: Time2 object time4 specified" & vbCrLf & _
Space(SPACING) & time6.ToUniversalString() )
End Sub
End Class
' Represents time and contains overloaded constructors.
Class CTime2
Inherits Object
Private mHour As Integer ' 0 - 23
Private mMinute As Integer ' 0 - 59
Private mSecond As Integer ' 0 - 59
' constructor initializes each variable to zero and
' ensures that each CTime2 object starts in consistent state
Public Sub New()
SetTime()
End Sub ' New
' CTime2 constructor: hour supplied;
' minute and second default to 0
Public Sub New(ByVal hourValue As Integer)
SetTime(hourValue)
End Sub ' New
' CTime2 constructor: hour and minute supplied;
' second defaulted to 0
Public Sub New(ByVal hourValue As Integer, _
ByVal minuteValue As Integer)
SetTime(hourValue, minuteValue)
End Sub ' New
' CTime2 constructor: hour, minute and second supplied
Public Sub New(ByVal hourValue As Integer, _
ByVal minuteValue As Integer, ByVal secondValue As Integer)
SetTime(hourValue, minuteValue, secondValue)
End Sub ' New
' CTime2 constructor: another CTime2 object supplied
Public Sub New(ByVal timeValue As CTime2)
SetTime(timeValue.mHour, timeValue.mMinute, timeValue.mSecond)
End Sub ' New
' set new time value using universal time;
' perform validity checks on data;
' set invalid values to zero
Public Sub SetTime(Optional ByVal hourValue As Integer = 0, _
Optional ByVal minuteValue As Integer = 0, _
Optional ByVal secondValue As Integer = 0)
' perform validity checks on hour, then set hour
If (hourValue >= 0 AndAlso hourValue < 24) Then
mHour = hourValue
Else
mHour = 0
End If
' perform validity checks on minute, then set minute
If (minuteValue >= 0 AndAlso minuteValue < 60) Then
mMinute = minuteValue
Else
mMinute = 0
End If
' perform validity checks on second, then set second
If (secondValue >= 0 AndAlso secondValue < 60) Then
mSecond = secondValue
Else
mSecond = 0
End If
End Sub ' SetTime
' convert String to universal-time format
Public Function ToUniversalString() As String
Return String.Format("{0}:{1:D2}:{2:D2}", _
mHour, mMinute, mSecond)
End Function ' ToUniversalString
End Class
Related examples in the same category