Do While loop through a Collection
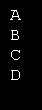
Imports System
Imports System.Collections
Public Class MainClass
Shared Sub Main(ByVal args As String())
Dim m_Employees As New Collection
m_Employees.Add(New Employee("A"))
m_Employees.Add(New Manager("B"))
m_Employees.Add(New Manager("C"))
m_Employees.Add(New Employee("D"))
Dim emp As Employee
Dim employee_enumerator As IEnumerator
employee_enumerator = m_Employees.GetEnumerator()
Do While (employee_enumerator.MoveNext)
emp = CType(employee_enumerator.Current, Employee)
Console.WriteLine( emp.Name )
Loop
End Sub
End Class
Public Class Employee
Public Name As String
Public Sub New(ByVal new_name As String)
Name = new_name
End Sub
Public Overridable Function IsManager() As Boolean
Return False
End Function
End Class
Public Class Customer
Public Name As String
Public Sub New(ByVal new_name As String)
Name = new_name
End Sub
End Class
Public Class Manager
Inherits Employee
Public Sub New(ByVal new_name As String)
MyBase.new(new_name)
End Sub
Public Overrides Function IsManager() As Boolean
Return True
End Function
End Class
Related examples in the same category