Loop through Collection
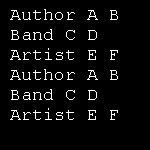
Imports System
Imports System.Collections
Public Class MainClass
Shared Sub Main(ByVal args As String())
Dim m_employees As New Collection
m_employees.Add(New employee("A", "B", "Author"))
m_employees.Add(New employee("C", "D", "Band"))
m_employees.Add(New employee("E", "F", "Artist"))
' Iterator.
Dim emp As employee
Dim employee_enumerator As IEnumerator
employee_enumerator = m_employees.GetEnumerator()
Do While (employee_enumerator.MoveNext)
emp = CType(employee_enumerator.Current, Employee)
Console.WriteLine(emp.Title & " " & emp.FirstName & " " & emp.LastName)
Loop
' For Each.
For Each emp1 As employee In m_employees
Console.WriteLine(emp1.Title & " " & emp1.FirstName & " " & emp1.LastName)
Next emp1
End Sub
End Class
Public Class Employee
Public FirstName As String
Public LastName As String
Public Title As String
Public Sub New(ByVal first_name As String, ByVal last_name As String, ByVal new_title As String)
FirstName = first_name
LastName = last_name
Title = new_title
End Sub
End Class
Related examples in the same category