Create a custom RoutedCommand, the CommandBinding objects, and the KeyBinding objects in code.
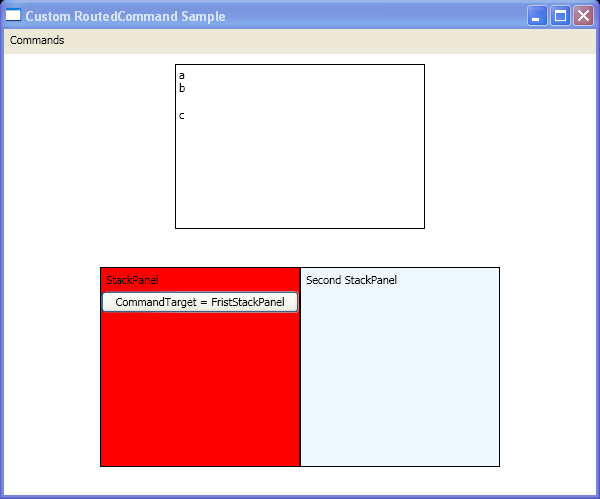
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:custom="clr-namespace:WpfApplication1"
Title="Custom RoutedCommand Sample"
Name="RootWindow" Height="500" Width="600"
Focusable="True">
<Window.CommandBindings>
<CommandBinding Command="{x:Static custom:Window1.ColorCmd}"
Executed="ColorCmdExecuted"
CanExecute="ColorCmdCanExecute"/>
</Window.CommandBindings>
<DockPanel>
<Menu DockPanel.Dock="Top" Height="25">
<MenuItem Header="Commands">
<MenuItem Header="Color Command" Command="{x:Static custom:Window1.ColorCmd}" />
</MenuItem>
</Menu>
<Border BorderBrush="Black" BorderThickness="1" Margin="10"
Height="165" Width="250" DockPanel.Dock="Top">
<TextBlock TextWrapping="Wrap" Margin="3">
a
<LineBreak/>
b
<LineBreak/>
<LineBreak/>
c
<LineBreak/>
</TextBlock>
</Border>
<StackPanel Orientation="Horizontal" HorizontalAlignment="Center" DockPanel.Dock="Bottom">
<Border BorderBrush="Black" BorderThickness="1" Height="200" Width="200">
<StackPanel Name="FirstStackPanel" Background="AliceBlue" Focusable="True">
<StackPanel.CommandBindings>
<CommandBinding Command="{x:Static custom:Window1.ColorCmd}" Executed="ColorCmdExecuted" CanExecute="ColorCmdCanExecute"/>
</StackPanel.CommandBindings>
<Label>StackPanel</Label>
<Button Command="{x:Static custom:Window1.ColorCmd}"
CommandParameter="ButtonOne"
CommandTarget="{Binding ElementName=FirstStackPanel}"
Content="CommandTarget = FristStackPanel" />
</StackPanel>
</Border>
<Border BorderBrush="Black" BorderThickness="1" Height="200" Width="200">
<StackPanel Background="AliceBlue" Focusable="True">
<Label>Second StackPanel</Label>
</StackPanel>
</Border>
</StackPanel>
</DockPanel>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Media
Imports System.Windows.Input
Namespace WpfApplication1
Public Partial Class Window1
Inherits Window
Public Shared ColorCmd As New RoutedCommand()
Public Sub New()
InitializeComponent()
End Sub
Private Sub ColorCmdExecuted(sender As Object, e As ExecutedRoutedEventArgs)
Dim target As Panel = TryCast(e.Source, Panel)
If target IsNot Nothing Then
If target.Background Is Brushes.AliceBlue Then
target.Background = Brushes.Red
Else
target.Background = Brushes.AliceBlue
End If
End If
End Sub
Private Sub ColorCmdCanExecute(sender As Object, e As CanExecuteRoutedEventArgs)
If TypeOf e.Source Is Panel Then
e.CanExecute = True
Else
e.CanExecute = False
End If
End Sub
End Class
End Namespace
Related examples in the same category