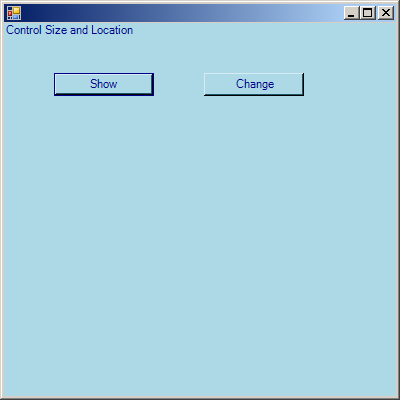
using System;
using System.Drawing;
using System.Windows.Forms;
public class FormClientSize : Form
{
private Button btnShow;
private Button btnChange;
private Label lbl;
public FormClientSize()
{
BackColor = Color.LightBlue;
ForeColor = Color.DarkBlue;
ClientSize = new Size(350,200);
Width = 350;
Height = 200;
btnShow = new Button();
btnShow.Location = new Point(50,50);
btnShow.Size = new Size(100,23);
btnShow.Text = "Show";
btnShow.Click += new System.EventHandler(btnShow_Click);
btnShow.Parent = this;
btnChange = new Button();
btnChange.Location = new Point(200,50);
btnChange.Size = new Size(100,23);
btnChange.Text = "Change";
btnChange.Click += new System.EventHandler(btnChange_Click);
btnChange.Parent = this;
lbl = new Label();
lbl.Text = "Control Size and Location";
lbl.Size = new Size(400,25);
lbl.Parent = this;
}
static void Main()
{
Application.Run(new FormClientSize());
}
private void btnShow_Click(object sender, EventArgs e)
{
Console.WriteLine("Button Bottom:" + btnShow.Bottom.ToString());
Console.WriteLine("Button Top:" + btnShow.Top.ToString() );
Console.WriteLine("Button Left:" + btnShow.Left.ToString() );
Console.WriteLine("Button Right:" + btnShow.Right.ToString() );
Console.WriteLine("Button Location:" + btnShow.Location.ToString() );
Console.WriteLine("Button Width:" + btnShow.Width.ToString() );
Console.WriteLine("Button Height:" + btnShow.Height.ToString() );
Console.WriteLine("Button Size:" + btnShow.Size.ToString() );
Console.WriteLine("Button ClientSize:" + btnShow.ClientSize.ToString() );
Console.WriteLine("Form Size:" + this.Size.ToString() );
Console.WriteLine("Form ClientSize:" + this.ClientSize.ToString());
}
private void btnChange_Click(object sender, EventArgs e)
{
this.Size = new Size(800,200);
}
}