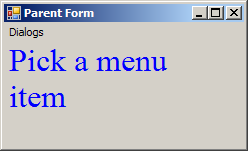
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class FormInvokeDialog : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
private System.Windows.Forms.MenuItem mnuModalBox;
private System.Windows.Forms.MenuItem menuItem1;
private System.Windows.Forms.MainMenu mainMenu1;
private string dlgMsg = "Pick a menu item";
public FormInvokeDialog()
{
InitializeComponent();
CenterToScreen();
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.menuItem1 = new System.Windows.Forms.MenuItem();
this.mnuModalBox = new System.Windows.Forms.MenuItem();
this.mainMenu1 = new System.Windows.Forms.MainMenu();
//
// menuItem1
//
this.menuItem1.Index = 0;
this.menuItem1.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.mnuModalBox});
this.menuItem1.Text = "Dialogs";
//
// mnuModalBox
//
this.mnuModalBox.Index = 0;
this.mnuModalBox.Text = "Show Modal Box";
this.mnuModalBox.Click += new System.EventHandler(this.mnuModalBox_Click);
//
// mainMenu1
//
this.mainMenu1.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.menuItem1});
//
// FormInvokeDialog
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(240, 105);
this.Menu = this.mainMenu1;
this.Name = "FormInvokeDialog";
this.Text = "Parent Form";
this.Resize += new System.EventHandler(this.FormInvokeDialog_Resize);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.FormInvokeDialog_Paint);
}
[STAThread]
static void Main()
{
Application.Run(new FormInvokeDialog());
}
protected void FormInvokeDialog_Resize (object sender, System.EventArgs e)
{
Invalidate();
}
protected void FormInvokeDialog_Paint (object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
g.DrawString(dlgMsg, new Font("times New Roman", 24),Brushes.Blue, this.ClientRectangle);
}
protected void mnuModalBox_Click (object sender, System.EventArgs e)
{
DialogForm myForm = new DialogForm();
myForm.Message = dlgMsg;
myForm.ShowDialog(this);
if(myForm.DialogResult == DialogResult.OK)
{
dlgMsg = myForm.Message;
Invalidate();
}
}
}
public class DialogForm : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
private System.Windows.Forms.Button btnCancel;
private System.Windows.Forms.Button btnOK;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.TextBox txtMessage;
public DialogForm()
{
InitializeComponent();
this.StartPosition = FormStartPosition.CenterParent;
}
private string strMessage;
public string Message
{
get{ return strMessage;}
set
{
strMessage = value;
txtMessage.Text = strMessage;
}
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.btnOK = new System.Windows.Forms.Button();
this.btnCancel = new System.Windows.Forms.Button();
this.txtMessage = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// label1
//
this.label1.Font = new System.Drawing.Font("Microsoft Sans Serif", 12F, System.Drawing.FontStyle.Bold);
this.label1.Location = new System.Drawing.Point(12, 8);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(240, 48);
this.label1.TabIndex = 1;
this.label1.Text = "Type in your message, and the main window will use it...";
//
// btnOK
//
this.btnOK.DialogResult = System.Windows.Forms.DialogResult.OK;
this.btnOK.Location = new System.Drawing.Point(16, 104);
this.btnOK.Name = "btnOK";
this.btnOK.Size = new System.Drawing.Size(96, 24);
this.btnOK.TabIndex = 2;
this.btnOK.Text = "OK";
this.btnOK.Click += new System.EventHandler(this.btnOK_Click);
//
// btnCancel
//
this.btnCancel.DialogResult = System.Windows.Forms.DialogResult.Cancel;
this.btnCancel.Location = new System.Drawing.Point(152, 104);
this.btnCancel.Name = "btnCancel";
this.btnCancel.Size = new System.Drawing.Size(96, 24);
this.btnCancel.TabIndex = 3;
this.btnCancel.Text = "Cancel";
//
// txtMessage
//
this.txtMessage.Location = new System.Drawing.Point(16, 72);
this.txtMessage.Name = "txtMessage";
this.txtMessage.Size = new System.Drawing.Size(232, 20);
this.txtMessage.TabIndex = 0;
this.txtMessage.Text = "";
//
// DialogForm
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(266, 151);
this.ControlBox = false;
this.Controls.Add(this.btnCancel);
this.Controls.Add(this.btnOK);
this.Controls.Add(this.label1);
this.Controls.Add(this.txtMessage);
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog;
this.MaximizeBox = false;
this.MinimizeBox = false;
this.Name = "DialogForm";
this.Text = "Some Custom Dialog";
this.ResumeLayout(false);
}
protected void btnOK_Click (object sender, System.EventArgs e)
{
strMessage = txtMessage.Text;
}
}