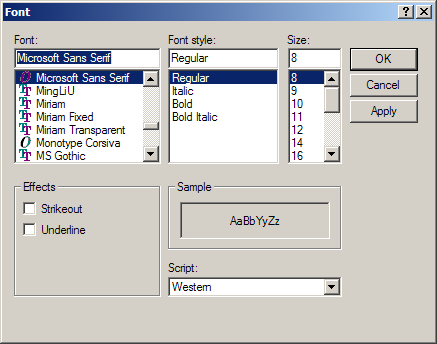
using System;
using System.Drawing;
using System.Windows.Forms;
public class FontDialogFontApplyEvent : Form
{
private Button btnChange;
private Label lbl;
private FontDialog fd;
public FontDialogFontApplyEvent()
{
Size = new Size(350,200);
btnChange = new Button();
btnChange.Location = new Point(200,50);
btnChange.Size = new Size(100,23);
btnChange.Text = "Change";
btnChange.Click += new System.EventHandler(btnChange_Click);
btnChange.Parent = this;
lbl = new Label();
lbl.Text = "test";
lbl.AutoSize = true;
lbl.Parent = this;
}
static void Main()
{
Application.Run(new FontDialogFontApplyEvent());
}
private void btnChange_Click(object sender, EventArgs e)
{
fd = new FontDialog();
fd.ShowHelp = false;
fd.ShowApply = true;
fd.Apply += new System.EventHandler(this.fd_Apply);
if (fd.ShowDialog() == DialogResult.OK)
lbl.Font = fd.Font;
}
private void fd_Apply(object sender, System.EventArgs e)
{
lbl.Font = fd.Font;
}
}