<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" Height="300" Width="300">
<StackPanel>
<ListBox FontSize="16" Height="150" Margin="5" Name="listBox1" SelectionMode="Extended">
<ListBoxItem>List Item 1</ListBoxItem>
<ListBoxItem>List Item 2</ListBoxItem>
<ListBoxItem>List Item 3</ListBoxItem>
</ListBox>
<StackPanel HorizontalAlignment="Center" Orientation="Horizontal">
<Label Content="_New item text:" VerticalAlignment="Center" Target="{Binding ElementName=textBox}" />
<TextBox Margin="5" Name="textBox" MinWidth="120" />
</StackPanel>
<StackPanel HorizontalAlignment="Center" Orientation="Horizontal">
<Button Click="btnAddListItem_Click" Content="Add Item" IsDefault="True" Margin="5" Name="btnAddListItem" />
<Button Click="btnDeleteListItem_Click" Content="Delete Items" Margin="5" Name="btnDeleteListItem" />
<Button Click="btnSelectAll_Click" Content="Select All" Margin="5" Name="btnSelectAll" />
</StackPanel>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System.Windows;
using System.Windows.Controls;
using System.Windows.Media;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void btnAddListItem_Click(object sender, RoutedEventArgs e)
{
if (textBox.Text.Length == 0)
{
MessageBox.Show("Enter text to add to the list.", Title);
}
else
{
ListBoxItem item = new ListBoxItem();
item.Content = textBox.Text;
item.IsSelected = true;
item.HorizontalAlignment = HorizontalAlignment.Center;
item.FontWeight = FontWeights.Bold;
item.FontFamily = new FontFamily("Tahoma");
listBox1.Items.Add(item);
textBox.Clear();
textBox.Focus();
}
}
private void btnDeleteListItem_Click(object sender, RoutedEventArgs e)
{
if (listBox1.SelectedItems.Count == 0)
{
MessageBox.Show("Select list items to delete.", Title);
}
else
{
while (listBox1.SelectedItems.Count > 0)
{
listBox1.Items.Remove(listBox1.SelectedItems[0]);
}
}
}
private void btnSelectAll_Click(object sender, RoutedEventArgs e)
{
listBox1.SelectAll();
}
}
}
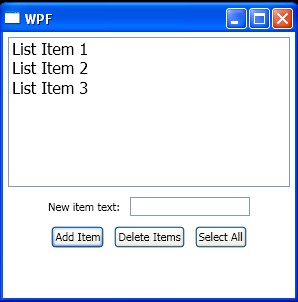