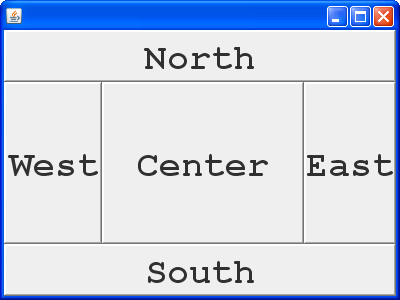
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.Insets;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JTextArea;
import javax.swing.JToolBar;
import javax.swing.border.EmptyBorder;
import javax.swing.border.LineBorder;
public class BorderLayoutExample {
public static void main(String[] args) {
JFrame f = new JFrame();
JMenuBar menubar = new JMenuBar();
JMenu file = new JMenu("File");
menubar.add(file);
f.setJMenuBar(menubar);
JToolBar toolbar = new JToolBar();
toolbar.setFloatable(false);
JButton bexit = new JButton(new ImageIcon("exit.png"));
bexit.setBorder(new EmptyBorder(0, 0, 0, 0));
toolbar.add(bexit);
f.add(toolbar, BorderLayout.NORTH);
JToolBar vertical = new JToolBar(JToolBar.VERTICAL);
vertical.setFloatable(false);
vertical.setMargin(new Insets(10, 5, 5, 5));
JButton selectb = new JButton(new ImageIcon("a.png"));
selectb.setBorder(new EmptyBorder(3, 0, 3, 0));
JButton freehandb = new JButton(new ImageIcon("b.png"));
freehandb.setBorder(new EmptyBorder(3, 0, 3, 0));
JButton shapeedb = new JButton(new ImageIcon("c.png"));
shapeedb.setBorder(new EmptyBorder(3, 0, 3, 0));
vertical.add(selectb);
vertical.add(freehandb);
vertical.add(shapeedb);
f.add(vertical, BorderLayout.WEST);
f.add(new JTextArea(), BorderLayout.CENTER);
JLabel statusbar = new JLabel(" Statusbar");
statusbar.setPreferredSize(new Dimension(-1, 22));
statusbar.setBorder(LineBorder.createGrayLineBorder());
f.add(statusbar, BorderLayout.SOUTH);
f.setSize(350, 300);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
}
}