BorderLayout is the default layout manager for the content pane of a JFrame, JWindow, JDialog, JInternalFrame, and JApplet.
- Place components against any of the four borders of the container and in the center.
- The component in the center fills the available space.
- You do not need specify all five areas of the container.
- The component in the north region takes up the entire width of the container along its top.
- South does the same along the bottom.
- The heights of north and south will be the preferred heights of the added component.
- The east and west areas are given the widths of the component each contains, where the height is whatever is left in the container after satisfying north's and south's height requirements.
- Any remaining space is given to the component in the center region.
- Constants used to specify areas: CENTER, EAST, NORTH, SOUTH, WEST
Its constructors:
public BorderLayout()
public BorderLayout(int hgap, int vgap)
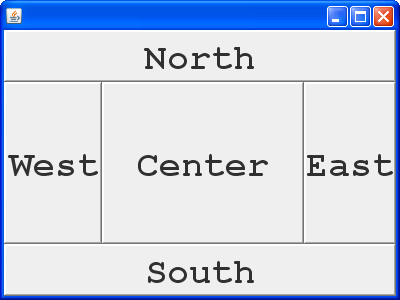
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.Font;
import javax.swing.BorderFactory;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.border.BevelBorder;
public class BorderLayoutExample extends JFrame {
public static void main(String[] args) {
BorderSample bs = new BorderSample();
bs.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Container pane = bs.getContentPane();
pane.setLayout(new BorderLayout());
JLabel label = new JLabel("North", JLabel.CENTER);
label.setFont(new Font("Courier", Font.BOLD, 36));
label.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
pane.add(label, BorderLayout.NORTH);
label = new JLabel("South", JLabel.CENTER);
label.setFont(new Font("Courier", Font.BOLD, 36));
label.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
pane.add(label, BorderLayout.SOUTH);
label = new JLabel("East", JLabel.CENTER);
label.setFont(new Font("Courier", Font.BOLD, 36));
label.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
pane.add(label, BorderLayout.EAST);
label = new JLabel("West", JLabel.CENTER);
label.setFont(new Font("Courier", Font.BOLD, 36));
label.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
pane.add(label, BorderLayout.WEST);
label = new JLabel("Center", JLabel.CENTER);
label.setFont(new Font("Courier", Font.BOLD, 36));
label.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
pane.add(label, BorderLayout.CENTER);
bs.setSize(400, 300);
bs.setVisible(true);
}
}