By changing the scrollOffset setting, you can control which part of the text field is visible.
To make sure that the end of the contents is visible, you need to ask the
horizontalVisibility property what the extent of the BoundedRangeModel is, to determine the width
of the range, and then set the scrollOffset setting to the extent setting, as follows:
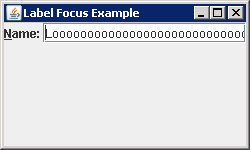
import java.awt.BorderLayout;
import java.awt.event.KeyEvent;
import javax.swing.BoundedRangeModel;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class LabelSampleBoundedRangeModel {
public static void main(String args[]) {
JFrame frame = new JFrame("Label Focus Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new BorderLayout());
JLabel label = new JLabel("Name: ");
label.setDisplayedMnemonic(KeyEvent.VK_N);
JTextField textField = new JTextField();
label.setLabelFor(textField);
panel.add(label, BorderLayout.WEST);
panel.add(textField, BorderLayout.CENTER);
frame.add(panel, BorderLayout.NORTH);
frame.setSize(250, 150);
frame.setVisible(true);
textField.setText("Loooooooooooooooooooooooooooooooooooooooooooooooooooooooong");
BoundedRangeModel model = textField.getHorizontalVisibility();
int extent = model.getExtent();
textField.setScrollOffset(extent);
System.out.println("extent:"+extent);
}
}