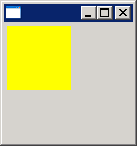
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.KeyEvent;
import org.eclipse.swt.events.KeyListener;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.graphics.GC;
import org.eclipse.swt.graphics.Rectangle;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Canvas;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class CanvasKeyEvent {
public static void main(String[] args) {
final Display display = new Display();
Shell shell = new Shell(display);
shell.setLayout(new RowLayout());
final Canvas canvas = new Canvas(shell, SWT.NULL);
canvas.setSize(500, 500);
canvas.setBackground(display.getSystemColor(SWT.COLOR_YELLOW));
canvas.addKeyListener(new KeyListener() {
public void keyPressed(KeyEvent e) {
GC gc = new GC(canvas);
Rectangle rect = canvas.getClientArea();
gc.fillRectangle(rect.x, rect.y, rect.width, rect.height);
Font font = new Font(display, "Arial", 32, SWT.BOLD );
gc.setFont(font);
gc.drawString("" + e.character, 15, 10);
gc.dispose();
font.dispose();
}
public void keyReleased(KeyEvent e) {
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
}
}