- Set the text from the cell into your editor's control when you create the control.
- Set the text from the control back into the cell any time it's modified.
Add Combo to Table as the Cell Editor and Exchange data between them
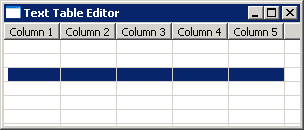
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.CCombo;
import org.eclipse.swt.custom.TableEditor;
import org.eclipse.swt.events.MouseAdapter;
import org.eclipse.swt.events.MouseEvent;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.graphics.Point;
import org.eclipse.swt.graphics.Rectangle;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Control;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Table;
import org.eclipse.swt.widgets.TableColumn;
import org.eclipse.swt.widgets.TableItem;
public class TableCellEditorComboDataExchange {
public static void main(String[] args) {
Display display = new Display();
final Shell shell = new Shell(display);
shell.setText("Text Table Editor");
shell.setLayout(new FillLayout());
final Table table = new Table(shell, SWT.SINGLE | SWT.FULL_SELECTION | SWT.HIDE_SELECTION);
table.setHeaderVisible(true);
table.setLinesVisible(true);
for (int i = 0; i < 5; i++) {
TableColumn column = new TableColumn(table, SWT.CENTER);
column.setText("Column " + (i + 1));
column.pack();
}
for (int i = 0; i < 5; i++) {
final TableItem item = new TableItem(table, SWT.NONE);
}
final TableEditor editor = new TableEditor(table);
editor.horizontalAlignment = SWT.LEFT;
editor.grabHorizontal = true;
table.addMouseListener(new MouseAdapter() {
public void mouseDown(MouseEvent event) {
Control old = editor.getEditor();
if (old != null)
old.dispose();
Point pt = new Point(event.x, event.y);
final TableItem item = table.getItem(pt);
if (item == null) {
return;
}
int column = -1;
for (int i = 0, n = table.getColumnCount(); i < n; i++) {
Rectangle rect = item.getBounds(i);
if (rect.contains(pt)) {
column = i;
break;
}
}
if (column != 1) {
return;
}
final CCombo combo = new CCombo(table, SWT.READ_ONLY);
for (int i = 0; i < 3; i++) {
combo.add("" + i);
}
combo.select(combo.indexOf(item.getText(column)));
editor.minimumWidth = combo.computeSize(SWT.DEFAULT, SWT.DEFAULT).x;
table.getColumn(column).setWidth(editor.minimumWidth);
combo.setFocus();
editor.setEditor(combo, item, column);
final int col = column;
combo.addSelectionListener(new SelectionAdapter() {
public void widgetSelected(SelectionEvent event) {
item.setText(col, combo.getText());
combo.dispose();
}
});
}
});
shell.pack();
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}