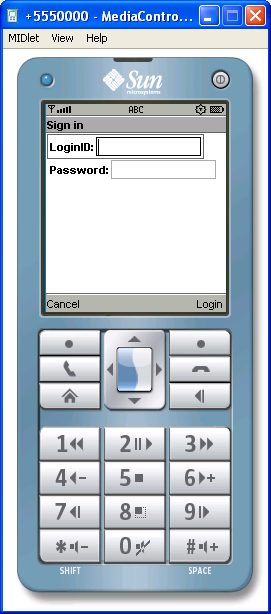
import javax.microedition.lcdui.Alert;
import javax.microedition.lcdui.AlertType;
import javax.microedition.lcdui.Choice;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Form;
import javax.microedition.lcdui.List;
import javax.microedition.lcdui.TextField;
import javax.microedition.midlet.MIDlet;
public class LoginMidlet extends MIDlet implements CommandListener {
private Display display;
private TextField userName = new TextField("LoginID:", "", 10, TextField.ANY);
private TextField password = new TextField("Password:", "", 10, TextField.PASSWORD);
private Form form = new Form("Sign in");
private Command cancel = new Command("Cancel", Command.CANCEL, 2);
private Command login = new Command("Login", Command.OK, 2);
public void startApp() {
display = Display.getDisplay(this);
form.append(userName);
form.append(password);
form.addCommand(cancel);
form.addCommand(login);
form.setCommandListener(this);
display.setCurrent(form);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
notifyDestroyed();
}
public void validateUser(String name, String password) {
if (name.equals("name") && password.equals("pass")) {
menu();
} else {
tryAgain();
}
}
public void menu() {
List services = new List("Choose one", Choice.EXCLUSIVE);
services.append("Check Mail", null);
services.append("Compose", null);
services.append("Addresses", null);
services.append("Options", null);
services.append("Sign Out", null);
display.setCurrent(services);
}
public void tryAgain() {
Alert error = new Alert("Login Incorrect", "Please try again", null, AlertType.ERROR);
error.setTimeout(Alert.FOREVER);
userName.setString("");
password.setString("");
display.setCurrent(error, form);
}
public void commandAction(Command c, Displayable d) {
String label = c.getLabel();
if (label.equals("Cancel")) {
destroyApp(true);
} else if (label.equals("Login")) {
validateUser(userName.getString(), password.getString());
}
}
}